Did you know that you can create a successful online course with WordPress?
Selling online courses is a popular online business idea that you can start with a very small investment and no technical knowledge.
Whether you’re looking to create an online course to sell or simply add an online course for your existing students, this tutorial is for you!
In this guide, we will show you how to easily create an online course using WordPress. We will also show you how to make money from it and make your online course a success.
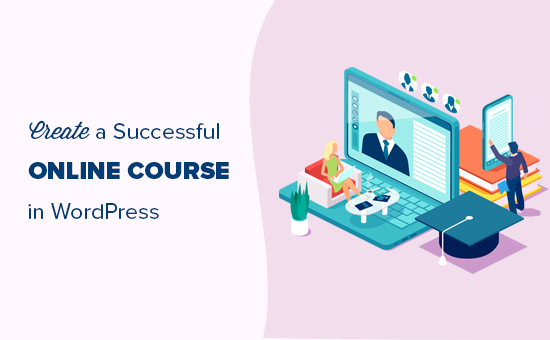
Here are the steps we will cover to help you create an online course with WordPress:
Ready? Let’s get started.
What Do You Need to Create / Sell an Online Course?
You will need the following things to create an online course.
- A course idea where you can help others learn new skills.
- A domain name. This will be your website’s address (Example, wpbeginner.com).
- A WordPress hosting account. This is where your website’s files are stored.
- An eLearning management add-on (also known as LMS plugin) to create and manage courses.
- Your undivided attention for the next 45 minutes.
You can build an online course with WordPress in less than an hour, and we’ll walk you through every step of the process.
Let’s get started.
Step 1. Setting up Your WordPress Website
There are plenty of website builders and online course platforms that you can use to build your own website. However, we always recommend WordPress because it offers you the maximum flexibility and freedom.
WordPress powers over 39% of all websites on the internet.
There are two types of WordPress, and beginners often end up confusing them.
First, there is WordPress.com which is a hosting service, and then you have the original WordPress.org also known as self-hosted WordPress. See our guide on the difference between WordPress.com vs WordPress.org.
We recommend using WordPress.org because it gives you access to all the WordPress features that you’ll need.
To start a self-hosted WordPress.org website, you’ll need a domain name ($14.99 / year), WordPress hosting ($7.99 / month), and SSL certificate to accept online payments ($69.99 / year).
This is quite a lot of startup money.
Luckily, Bluehost, an officially recommended WordPress hosting provider, has agreed to offer our users a free domain name, free SSL certificate, and a 60% discount on web hosting. Basically, you can get started for $2.75 per month.
→ Click Here to Claim This Exclusive Bluehost Offer ←
After purchasing hosting, head over to our guide on how to create a WordPress website for step by step set up instructions.
Step 2. Install and Setup MemberPress LMS Plugin
Now that your WordPress website is ready, the next step is to install and setup a Learning Management System add-on. This will allow you to create your online course and add it to your website.
First, you need to install and activate the MemberPress plugin. For more details, see our step by step guide on how to install a WordPress plugin.
MemberPress is the best LMS plugin for WordPress. It is an all-in-one solution with complete course management, lesson plans, subscriptions, access control, payment management, and more.
Course creators around the world use MemberPress to create profitable courses and earn over $400 million dollars every year.
Upon activation, you need to visit MemberPress » Settings page to enter your license key. You can find this information under your account on the MemberPress website.
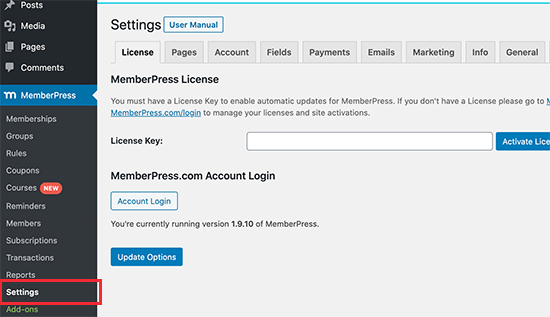
Once you have entered the information, click on the ‘Activate License Key’ button to store your settings.
Next, you need to switch to the ‘Payments’ tab and click on the (+) add button to set up a payment gateway. MemberPress supports PayPal and Stripe (Authorize.net support included in Pro and Plus plans).
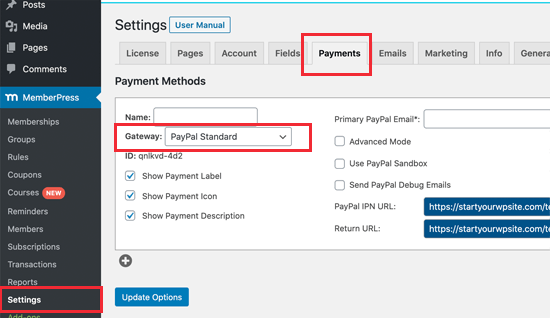
Simply select your payment gateway and then fill in the required information. You can also set up multiple payment methods by clicking on the (+) button again and repeat the process.
Don’t forget to click on the ‘Update Options’ button to save your payment settings.
Step 3. Creating Your First Course
The course creation process in MemberPress makes it super easy to create and manage online courses. It comes with a very easy to use course builder that allows you to create courses, add sections, edit lessons, and more.
First, you need to visit MemberPress » Courses page where you’ll see a button to install and activate the courses addon.
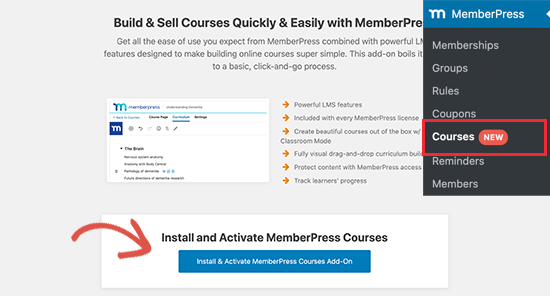
Clicking on it will automatically install and activate the MemberPress courses addon, and you’ll be redirected to the courses page.
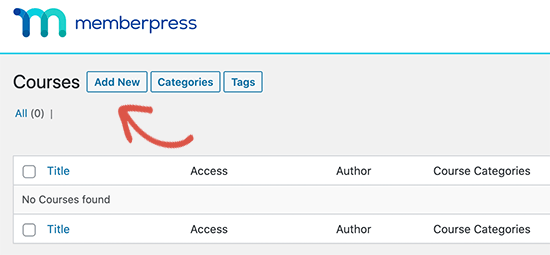
Simply click on the ‘Add New’ button at the top to create your first course. This will launch the MemberPress course builder screen.
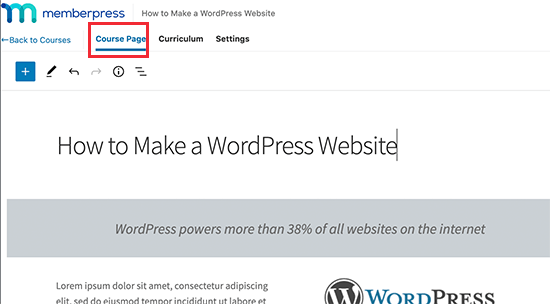
First, you’ll see the ‘Course Page’ where you need to provide a course topic, title, and description. MemberPress course builder uses the default WordPress block editor, so you can get creative and make an impressive course page layout.
You can also add ‘Course Categories’ and ‘Course Tags’, set a featured image, and provide a course page excerpt under the course page settings.
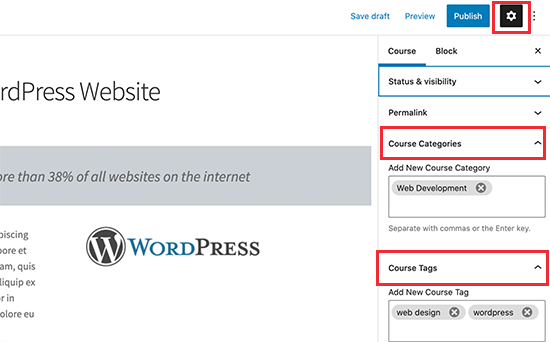
Step 4. Adding Sections and Lessons to Your Course
MemberPress also makes it easy to quickly start adding course contents for each course without switching to a different page.
Simply switch to the ‘Curriculum’ tab and click on the Add Section button to create your course outline.
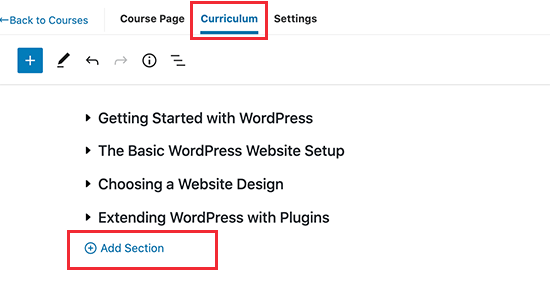
You need to provide a title for a section and then click on the Add Lesson button under the section to add lessons.
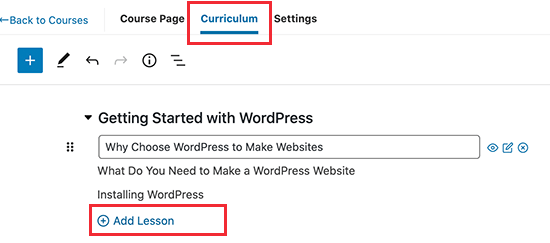
After adding a lesson, you can start adding lesson content by clicking on the edit button next to each lesson.
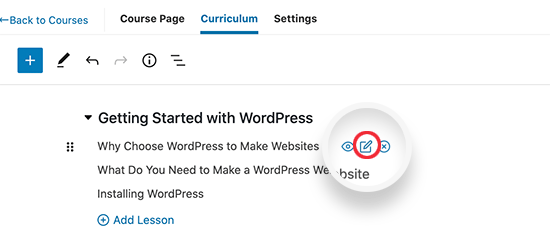
You will be asked to save your changes after that your lesson will open up with the familiar block editor screen.
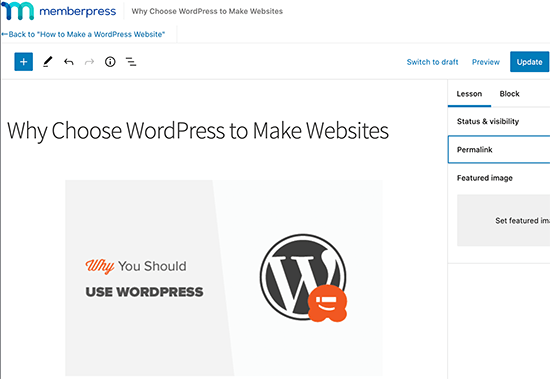
You can add your lesson content here with the full advantage of the block editor. This allows you to upload images, embed videos, add text, offer downloadable digital products such as powerpoints, PDF eBooks, actionable worksheets, and other course materials.
Don’t forget to click on the Update button to save your lesson. You can return back to the Course by clicking on the ‘Back’ link at the top of the editor.
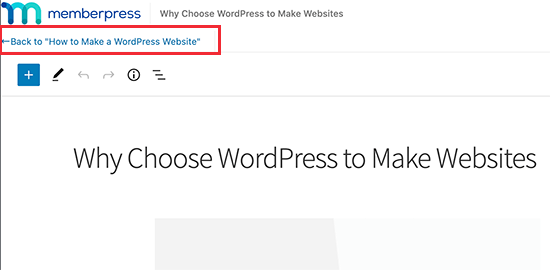
Repeat the process to add more lessons to your course.
Once you are finished adding course content, don’t forget to click on the ‘Publish’ button to make your course accessible to eligible users.
Step 5. Creating Course Membership Subscriptions
MemberPress allows you to easily sell online courses with subscription plans. You can create as many membership plans as you like, and users can select a plan to pay for your online course.
You can also sell all your courses under single membership, offer free courses, or you can add different courses for each plan. This depends on how you plan to structure your sales funnel.
A lot of people use a combination of free courses and paid courses to build their audience and maximize passive income.
To add a membership, simply go to MemberPress » Memberships page and click on the Add New button.
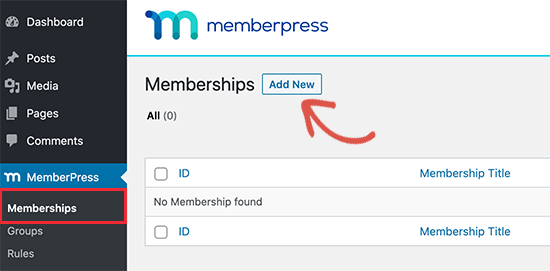
Next, you will reach the create new membership page. From here, you first need to provide a title for this membership plan and then add some description.
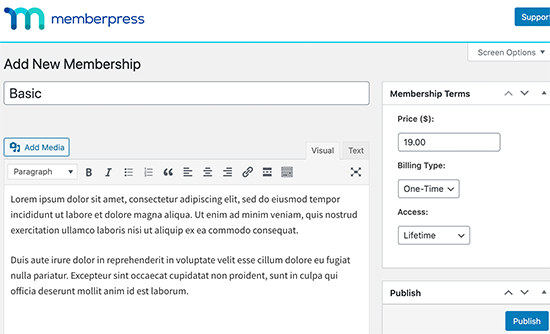
After that, you can enter the membership pricing under ‘Membership Terms’ box on the right. You can also choose the access duration from lifetime, expire (recurring), or fixed expire.
Next, you need to scroll down to the Membership Options section. This where you can configure advanced membership options like sign up button, welcome email, pricing box, and more.
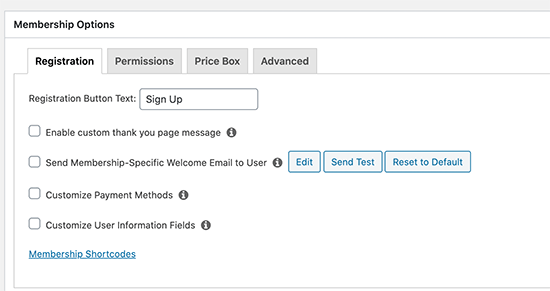
Once you are finished, you can click on the Publish button to save your changes.
Repeat the process if you need to create more membership plans.
Step 6. Restrict Course Access to Membership Plans
The best part about using MemberPress is its powerful access control rules. They allow you to decide who gets access to your online course.
Simply, go to MemberPress » Rules page and click on the ‘Add New’ button.
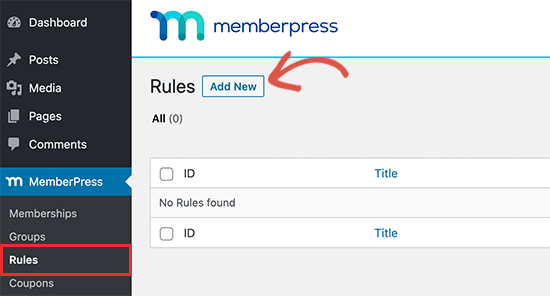
This will bring you to the Rule wizard page. First, you need to select the content you want to protect under the ‘Protected’ content section.
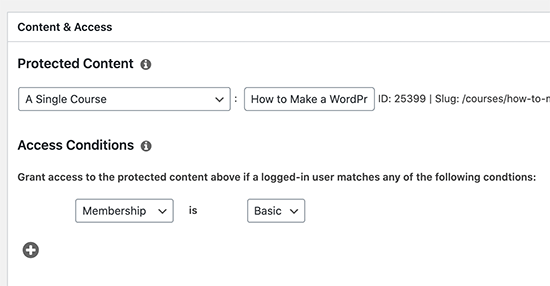
For instance, here we have chosen a single course under the protected content.
Below that, you need to select the conditions that need to be matched for users to access that content. For instance, we have chosen our membership plan here.
Don’t forget to click on the ‘Save Rule’ button to save your settings.
Step 7. Adding a Link to Course Sign up and Register Page
MemberPress makes it easy to easily send users to the page where they can register and sign up for your course by purchasing a membership plan.
It automatically generates a link for each membership plan that you can add anywhere on your site.
Simply edit a membership plan and you’ll see the link below the membership title. You can also manually type in your domain name followed by /register/your-membership-title/
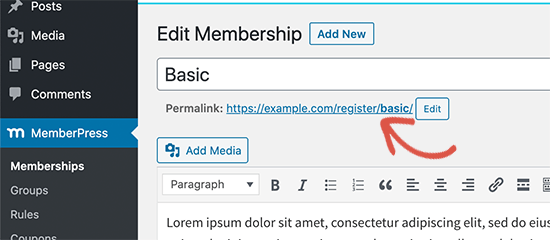
You can add this link anywhere on your website in a post, page, or navigation menu and it will take users to the registration page.
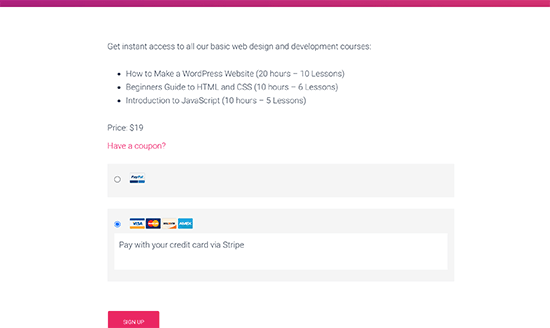
You can even share this link in your sales pages, webinars, podcasts, Facebook group, social media, and other traffic channels.
Pro Tip: We recommend using PrettyLinks to create memorable short links to share in podcasts, webinars, and social media.
Step 8. Preview & Customize Your Online Course
MemberPress allows you to use the classroom mode by default, which means your course pages and content will always look good regardless of which WordPress theme you are using.
You can simply go to view a course by visiting:
https://example.com/courses/
Don’t forget to replace the example.com with your own domain name.
You’ll see all your courses listed there. You can click on the ‘Preview as’ menu to see how it would look to the logged out users.
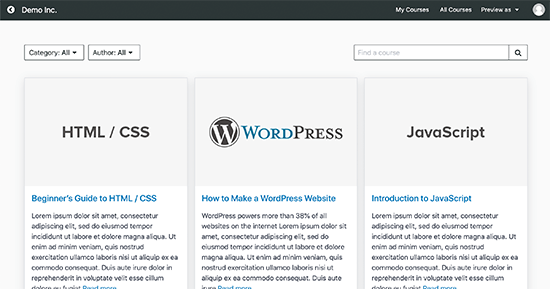
Clicking on a course will open the course, and you will be able to see course overview, sections, and lessons. It is super easy to navigate and also keeps tracks of user’s progress so that they can continue where they left off.
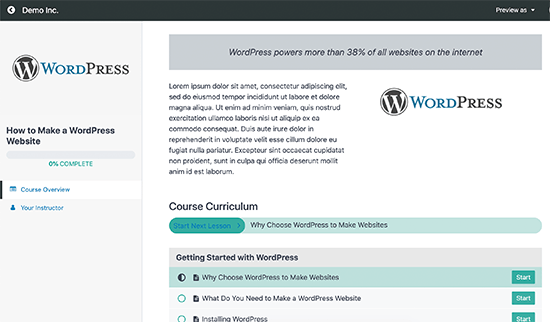
You can also customize the course page templates by visiting Appearance » Customize page and clicking on the ‘MemberPress Classroom’ tab.
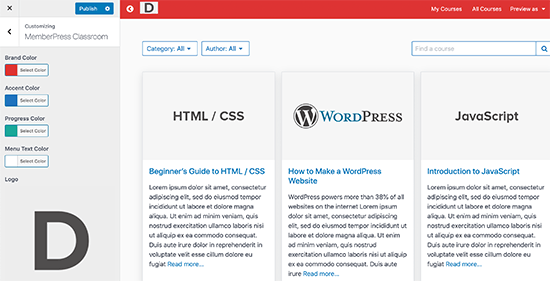
Here, you can upload your brand logo and change colors to match rest of your website.
Don’t forget to click on the Publish button to save your changes.
Step 9. Selling Your Online Course with More Powerful Features
Both MemberPress and WordPress are super flexible. This allows you to use them with any other tools to grow your business and reach more users.
For example, you can create a powerful membership site / community that offers paid content and perks along with courses.
You can also use MemberPress with other LMS plugins like LearnDash. This allows you to use LearnDash for course creation and use MemberPress for powerful subscription, payments, memberships, and other features.
Alternatively, if you want to sell other items like physical goods related to your course, swags, etc, then you can use WooCommerce to manage payments and orders. This will let you build a proper online store for your website.
The other advantage of WordPress + MemberPress combo is that it also makes it easier for you to promote your online course and make money online.
Let’s take a look at few ways to promote your online course, attract target audience, and make it successful.
1. Create Landing Pages for Your Online Courses
Your WordPress theme would be able to help you create a highly engaging website. However, you may need to quickly create landing pages to describe course details, showcase instructors, highlight special offers, etc.
Custom landing pages and sales pages are proven to increase course sales.
We recommend using SeedProd. It is the best WordPress page builder and allows you to create professional landing page layouts without writing any code.
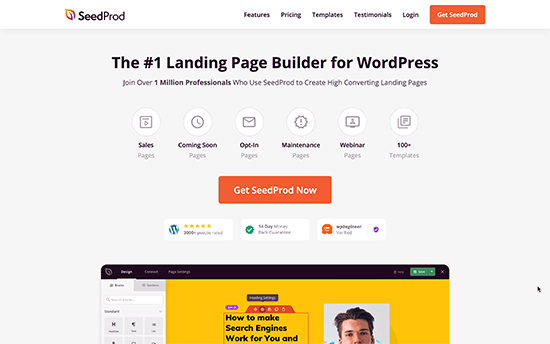
For detailed instructions, see our guide on how to create a custom page in WordPress.
2. Learn The SEO Basics
Search engines are the #1 traffic source for most websites on the internet. This is why you’ll need to learn how to make your online course website rank higher in search engines.
With the help of WordPress plugins and some basic SEO best practices, you’d be easily able to compete with the big guys.
We now recommend users to use All in One SEO for WordPress plugin.
To learn more, see our complete WordPress SEO guide for beginners with step by step instructions.
3. Track Marketing Data
A lot of beginners develop their marketing strategy based on guesswork. You don’t have to do that when you can get actual data to make informed decisions.
For that, you’ll need MonsterInsights. It helps you install Google Analytics and see human-readable reports inside your WordPress dashboard.
You can see where your visitors are coming from, what they do on your website, your most popular pages, and more. You can then improve your website to improve your conversions and boost sales.
4. Start Building an Email List
After a while, you would notice that most visitors who come to your website don’t sign up for your online course. The problem is that you would not be able to reach out to those users once they leave your website.
To address this, you need to start an email newsletter. This way you would be able to collect email addresses and reach out to those users and bring them back to your website.
We recommend using Constant Contact or ConvertKit for email marketing.
If you’re looking for alternatives, see our comparison of the best email marketing services.
5. Convert Website Visitors into Subscribers and Customers
Most visitors who come to your website will leave without enrolling into your online course. This is why it’s important to convert those abandoning visitors into subscribers or paying customers.
This is called conversion optimization.
The best tool for the job is OptinMonster. It is the best conversion optimization software on the market and helps you grow your business with more leads and sales.
For more details, see our guide on how to convert website visitors into customers.
Need even more tools? See our complete list of the best tools to grow your WordPress website like a total pro.
Frequently Asked Questions about Creating an Online Course (FAQs)
Over the last 10 years, we have helped thousands of entrepreneurs create their own online course. Below are the answers to the most frequently asked questions about creating an online course.
How can I create high-quality videos for my course?
Videos are an important element of online courses. It helps you better demonstrate your subject matter expertise and connect with your audience.
When first starting out, you don’t need to invest in fancy video equipments. A good Ultra HD webcam like Logitech Brio is sufficient for most users.
For screencasts and screen recording, you can use Camtasia or Screenflow for Mac.
Recently, our team has started using Descript online video editing platform, and it can significantly improve your workflow.
Can I use other online learning platforms with WordPress?
There are many online course platforms that you can use to build and sell courses.
This includes Teachable, Thinkific, Kajabi, Udemy, etc.
You can use any of them alongside your WordPress website. Depending on your needs, these platforms may offer an easier online course creation solution.
However they’re generally more expensive and/or take a revenue share from your course sales.
Which is the best webinar platform for course creators?
Nothing beats live webinars when it comes to online education. You can use to boost your audience engagement and improve membership retention.
We have compared the best webinar platforms here.
Most of these solutions will let you create live webinars, automated evergreen webinars, and come with tons of webinar engagement features.
How can I validate my course idea?
Creating online course content takes a lot of time and effort. This is why we always recommend users to validate their course idea before hands.
There are several ways to validate a course idea.
You can run a poll or survey on your website, ask for audience feedback on social media, or look at your most popular blog posts or YouTube videos because often the most popular ones can be turned into courses.
What’s the one “little-known” thing that I can do to make my online course successful?
While there are many tactics that you can use to make your online course idea successful and profitable.
The little-known tactic that works really well is case studies. Unfortunately not enough course creators use them.
A case study allows you to highlight the most successful students in your community. This not only provides encouragement to others, but it can also help those who don’t know how to take maximum advantage of your products.
We use case studies in many of our own businesses for social proof to boost conversions, but also to help our new users find encouragement and motivation.
We hope this article helped you easily create a successful online course in WordPress. You may also want to see our tips on how to add push notifications to connect with visitors after they leave your website, our our comparison of best live chat software for membership sites.
If you liked this article, then please subscribe to our YouTube Channel for WordPress video tutorials. You can also find us on Twitter and Facebook.
The post How to Create and Sell Online Courses with WordPress (Step by Step) appeared first on WPBeginner.