I want to create button delete all records in tables database with php
My WordPress Site Was Hacked! What Can I Do to Fix It and Prevent It From Happening Again?
WordPress is the most popular platform for websites. However, it is also the most compromised CMS on the web due to the sheer number of websites. Even if you have basic protection on your website,...
The post My WordPress Site Was Hacked! What Can I Do to Fix It and Prevent It From Happening Again? appeared first on 85ideas.com.
5 Ways to Sort Wisely
Hey to everyone! I guess you all did sorting at least once. I also guess that everyone knows a sorting called "Bubble," cause it is the easiest and most popular. But when it comes to time and your memory, what will you do?
Imagine a situation. You have a mass that contains about 10^100 elements. Is it still good to use bubble sorting? Just imagine checking every element... and not once. It is not so difficult to count how many operations it is and how much time it takes.
What is most effective social media platform for paid campaign?
Which one is the best for paid campaigns and gain best results?
Facebook, Twitter, Instagram, Pinterest, LinkedIn, TikTok or any other better option if possible.
miss rose makeup
please told me the technique that create higher rank of mine website through key words just miss rose makeup is cosmetic website
How to Create Google Web Stories in WordPress
Recently, Google updated its official Web Stories WordPress plugin. This plugin allows website owners to embed their content into Google search results, an important thing to consider when managing how your company website is presented online. The Web Stories plugin makes robust content creation tools accessible for WordPress website owners. You can now generate Web […]
The post How to Create Google Web Stories in WordPress appeared first on WPExplorer.
Why IT Outsourcing Is The Best Strategy In Post-Covid Times?
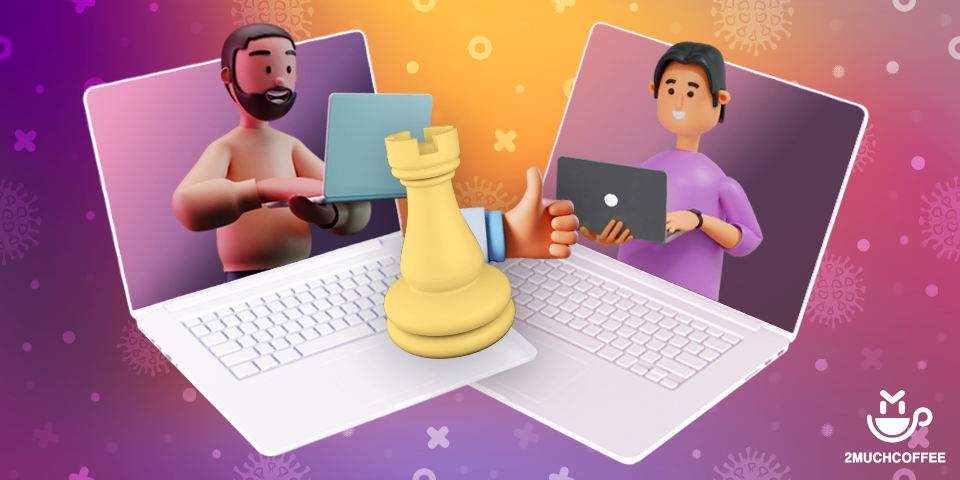
The IT outsourcing industry is a flourishing and growing field that helps businesses cut costs and scale up their services while working remotely. At present, quite an extensive number of companies have realized the benefits of outsourcing internal IT projects to remote dedicated teams.
Due to this, there is a lot of demand for remote software development services. IT outsourcing firms now work endlessly to fulfill clients' needs because businesses are adopting cloud services and their applications to scale up, connect, and collaborate with their employees and customers.
According to Gartner, as many as 88% of the companies worldwide have introduced the possibility of work from home after COVID-19 was declared a pandemic. As it turned out, hiring remote employees is an excellent idea. And if you are wondering why — we invite you to read this article.
How COVID-19 changed the rules for software development?
According to McKinsey Global Survey, COVID-19 has speeded the adoption of digital technologies by several years. The pandemic forced entrepreneurs to introduce new strategies and practices, allowing them to operate freely without the need for face-to-face meetings. The key changes include mainly:
1. Increase the importance of technology and the use of modern tools
First of all, to work efficiently in remote teams, companies must use appropriate tools. This applies to solutions to:
communication (e.g. online instant messaging);
online meetings, including remote onboarding integration of new employees;
signing contracts (use of electronic signature etc.);
tracking working time;
task and project management;
analytics and data visualization; and many others.
2. Reduce the geographical distance between customers and business partners
Moreover, also meetings with clients and business partners took the form of online meetings, which significantly reduced the importance of geographical distance. This new situation made the companies more open to cooperation with foreign companies because nowadays, the geographical location of the contractor is no longer important. It is confirmed by the results of the above-mentioned study, according to which companies confirmed that at least 80% of their customer interactions today are digital in nature.
3. Wider choice of employees and business partners
The aforementioned reduction of the importance of the employee's or partner's location has resulted in companies having much greater possibilities to establish valuable cooperation. This is particularly important in the case of experienced software developers who are still hard to find on the labor market. Thanks to the possibility of remote cooperation, the entrepreneurs can find specialists with a profile and skillset perfectly suited to their company's needs.
Why remote software development is the future?
Speeded digitalization caused by pandemic showed that remote software development is the future of product development. Why? There are several reasons for this.
1. Huge talent pool
The first and most important advantage that makes remote software development the future of product development is, of course, unlimited possibilities of searching for new employees. As an employer, you don't have to limit the search for employees from the nearest area — you can search for them all over the world!
According to a McKinsey survey, 87% of organizations are experiencing or expecting to face a tech talent shortage within the next few years.
The solution is to look for remote employees, especially in countries where the shortage of programmers is relatively low. A good direction is Ukraine, which has 20,000 IT specialists and provides around 16,000 IT graduates per year.
2. Costs reduction
Hiring a remote IT team also reduces costs. Product owners can look for service providers from the regions where rates are more competitive.
However, the lower costs of an employee do not necessarily mean lower quality. Offering a remote job offers a better chance to find talented specialists on the market. For example, if you decide to look for employees in Central and Eastern Europe, you can work with some of the best programmers in the world (the confirmation you will find in point 6).
3. Speed up the recruitment process
As an employer, you surely know how difficult it is to find a good and qualified IT specialist. Recruitment processes take months, which also generates high costs and frustration for HR departments. Expanding the search to other countries is a step that will allow you to shorten the process of searching for new candidates and make HR departments' workers easier.
4. Improve efficiency
According to the CoSo Cloud Survey, as many as 77% of people who work remotely claim that they're more productive during working from home. People are focused on their tasks, have fewer distractions, and what's more, they want to show that they perform their duties properly. These results show that remote working is the future of many sectors, not just IT.
5. Maintain a competitive advantage
If you don't decide to hire remote developers, you risk losing your competitive advantage. Nowadays, most digital companies choose this option because it is a very important issue for employees who just enjoy working remotely and can't imagine returning to the office. After a pandemic, remote work opens many opportunities, such as working from any place in the world. Lack of such an opportunity in your organization may result in big problems with finding valuable employees.
6. Access to world-class developers
Do you want to find world-class software developers who will help you build a top-quality product? If you decide to hire remote workers, you will have this opportunity.
As we mentioned before, one of the best directions both to outsource IT services and hire software developers is Central and Eastern Europe. Why? For example, according to Hackerrank, software developers in Ukraine are in eleventh place among the most skilled software engineers in the world. If you decide to build your software remotely, you will have the opportunity to work with the best specialists from this country without the need to offer them relocation.
7. Create a diverse work environment
Nowadays, building a culture of diversity is extremely important. A remote team consisting of employees located in different countries can learn a lot from each other with valuable tips. It is also a factor that shows that you are an open and forward-thinking employer who is focused on development and innovation.
8. Perfect choice for small and medium-sized companies
If you are a start-up owner, you should consider outsourcing your software development services. This is a great option if you need the support of experienced software developers in specific product development.
Currently, depending on your company’s location and direction of hiring, you will probably encounter two terms: offshoring and nearshoring.
Offshoring is one of the most budget-cutting methods of outsourcing. It means outsourcing the processes to vendors from distant countries, such as India or China, where the talent pools are vast, and the expenses are low. The biggest challenge of this option is the timezone as well as a cultural difference.
Nearshoring means outsourcing to a nearby country. This option allows holding face-to-face meetings more frequently, at a lower cost. Moreover, cultural compatibility is higher, which facilitates work coordination and reduces the risk of misunderstanding.
How to make remote software development work?
Remote working is a prominent part of the work at 2muchcoffee. Our employees can work from the office and remotely - depending on their preferences. Our team works effectively, which is proved by a significant number of satisfied customers. So, what is our recipe for effective remote working? Here are some tips that may be useful for your company.
Use of the best tools for remote teams
The key to effective remote working is good tools that facilitate processes such as this:
communication in a team,
onboarding new employees,
workflow,
classification and status of the tasks.
Regular online meetings
In remote teams, clear and regular communication is essential. That is why we meet every day for 15-minutes daily meetings, during which we talk about issues that come up in a project. The principle is simple - it has to be short and concise.
Building a culture of trust and support
Remote working, especially for beginners, is a real challenge. To help them find their way in the new reality, you need the support of more experienced colleagues. At Ideamotive, we make sure that every employee feels an important part of the team, in which we trust and support each other.
Final Thoughts
Switching to remote work was associated with many challenges. However, as it turned out, it was a much-needed change, which gave many new opportunities and made software development in a remote team almost mandatory.
If you're looking for a software developers team that will be perfectly matched with your industry, technology, team dynamics, and company culture - contact us so we can answer all of your questions.
Must Have Tools for Dev Teams to Work Remotely and Get the Most Out of It
There is a growing need for employees working online from the comfort of their own home or from any other place that suits them more than a classic office. Such employees are not under the...
The post Must Have Tools for Dev Teams to Work Remotely and Get the Most Out of It appeared first on 85ideas.com.
How to Move a Site from WordPress Multisite to Single Install
Do you want to move a site from a WordPress multisite to a single install?
If you run a WordPress multisite network, sometimes, you may need to move one of the websites to its own separate WordPress install.
In this article, we’ll show you how to easily move a site from WordPress multisite to its own single install while preserving SEO rankings and all your content.

Step 1: Getting Started
To move a website from a WordPress multisite network, you’ll need a domain name.
If you already have a domain name where you want to install the single site, then you are good to go.
If you don’t have a separate domain name, you’ll need to register and add a new domain name to your hosting account.
We recommend using Domain.com. They are one of the best domain name registrars in the world and offer beginner-friendly domain management experience.
For more details, see our article on how to register a domain name.
Alternatively, you can buy a separate hosting account and domain name for your fresh WordPress install.
We recommend using Bluehost. They are offering a free domain name with a generous discount on hosting.
Basically, you can get started for $2.75 per month.
After getting your domain name and hosting, the next step is to install WordPress.
See our step-by-step WordPress installation tutorial if you need help.
Important: Since you are going to make some serious changes to your WordPress multisite, it is necessary to create a complete WordPress backup before you do anything else.
Now that everything is set up, let’s move a site from WordPress multisite network to its single install.
Step 2: Exporting a Single Site in WordPress Multisite Network
The built-in WordPress import/export functionality works the same way in multisite as it does on a single site install. We will use the default tools to export the data from a site on a WordPress multisite network.
First, you need to log in to the dashboard of the single site you want to move, and then click on Tools » Export.
Next, you want to ensure all content is checked and click on the Download Export File button.

WordPress will now create an XML file containing all your data and send it to your browser for download.
Be sure to save the file on your computer because you will need it later.
Step 3: Importing Child Site to New Domain
Login to the WordPress admin area on the new location where you want to move your child site and then go to Tools » Import. On the import screen, WordPress will show you a number of import options.

You need to click on the ‘Install Now’ link below ‘WordPress.’
Wait for the importer to be installed and then click on the ‘Run Importer’ link.

On the next screen, you will be asked to upload the WordPress export file you downloaded earlier from the WordPress multisite.
Click on the Choose file button to select the file from your computer and then click on the ‘Upload file and import’ button.

On the next screen, WordPress will ask if you would also like to import users. If you do nothing, then WordPress will import all users. This is recommended if you do not want to change authors.
You will also see the Import Attachments option, and you want to make sure it is checked so that WordPress can download images from your posts and pages.
Don’t worry if it misses out on some or most of your images. You can import them separately afterward.

Click on the ‘Submit’ button to continue.
WordPress will now start importing your content. This will take a few minutes depending on how much content you have. Once it is done, you will see a notification that says ‘All done. Have fun!’

That’s all. You have successfully imported data from a multisite network child site to an individual WordPress install. There are still a few things left to do.
Step 4: Setting up Redirection
If you were using WordPress multisite with custom domains, then you don’t have to set up any redirection.
However, if you were using subdomains or directory structures in your WordPress multisite, you need to set up redirection so that users coming to your old URLs are redirected to your new site.
There are two ways to do this. You can set up a redirect using a WordPress plugin (recommended), or you can add some code to your WordPress .htaccess file.
We’ll show you both methods, and you can choose the one that best suits you.
Note: Make sure that your old site on the multisite network and the site on the new domain are both using the same permalink structure.
Method 1. Setting Redirects Using All in One SEO for WordPress
This method is easier and recommended for all users. We’ll be using All in One SEO for WordPress, which is the best WordPress SEO plugin on the market.
It allows you to easily optimize your WordPress site for search engines and comes with powerful features like SEO analysis, custom XML sitemaps, Schema.org support, and a redirects manager.
First, install and activate the All in One SEO for WordPress plugin on your WordPress multisite and then Network Activate it for the child site. For more details, see our guide on network activating plugins on WordPress Multisite.

Note: You’ll need at least the Pro plan to access the Redirection Manager feature.
Next, you need to install and network activate the Redirection Manager addon. You can find it under ‘Downloads’ from your account page on the All in One SEO website.

Once you have network-activated both plugins, you need to switch to the dashboard of the child site.
From here, go to the All in One SEO » Redirects page and switch to the ‘Full Site Redirect’ tab.

First, turn on the Relocate Site option by toggling the switch next to it.
Then, enter your new site’s domain name next to ‘Relocate to domain’ option.
Now click on the Save Changes button to store your settings.
All in One SEO for WordPress will now redirect users to your new domain name.
Method 2. Setting up Redirects using the Redirection Plugin
First, you need to install the Redirection plugin on your WordPress Multisite.
You can Network Activate a plugin, or you can log in as Super Admin on your child site and activate the Redirection plugin for that particular site alone.
After that, you need to visit the admin dashboard of the child site for which you want to set up the redirect.
Redirecting from Subdomain to New Domain
The Redirection plugin makes it super easy to point a domain name to a different one.
Simply go to the Tools » Redirection page and switch to the ‘Site’ tab.

Simply enter your new domain name and then click on the ‘Update’ button to save your settings.
The plugin will redirect all your site users to your new domain name with the correct permalink structure.
The advantage of this method is that you can still log in to the admin area of your old subdomain.
Redirecting from Directory to New Domain
If your multisite uses a directory-based URL structure, then the Redirection plugin makes it easy to redirect it properly to your new domain.
Simply go to the Tools » Redirection page on your sub-site, and then click on the Add New button at the top.

This will take you to Redirection’s setup form. Here is how you need to fill in that form:
Source URL: ^childsite/(.*)$
Target URL: https://example.com/$1
Be sure to replace childsite and example.com with the name of your subsite and its new location.
Don’t forget to change select ‘Regex’ from the dropdown to the right, and then just click on the Add Redirect button to save your settings.

You can now visit your sub-site to see the redirects in action.
Method 2. Setting up Redirects Using .htaccess file
For this method, you need to add redirect rules to the .htaccess file in your WordPress hosting account for your multisite network.
Subdomain to New Domain Redirect
For subdomain installs, you need to use this code in the .htaccess file of your WordPress multisite.
Options +FollowSymLinks
RewriteEngine on
RewriteCond %{HTTP_HOST} ^subdomain.example.com$ [NC]
RewriteRule ^(.*)$ http://www.example.net/$1 [L,R=301]
This code redirects visitors coming to any page on subdomain.example.com
to http://www.example.net
. The $1
sign at the end of the destination URL ensures that your users land on the same page they requested.
Redirecting From Directory to New Domain
For directory-based multisite installs, you’ll need to paste the following code in the .htaccess file of your WordPress multisite.
Options +FollowSymLinks
RewriteEngine On
RewriteRule ^childsite/(.*)$ http://example.net/$1 [R=301,L]
This code simply redirects any users coming on http://www.example.com/childsite/
to http://example.net
. The $1 makes sure that your users land on exactly the same page or post they requested.
Don’t forget to replace childsite and example.net with the name of your subsite and its new location.
Step 5: Troubleshooting the Migration
Moving a site is not a routine task, so it is likely that you may come across some issues.
1. Export File Too Large – If your WordPress export file is too large, you may fail to import it properly. To fix this, you may need to split large XML file into smaller pieces.
2. Images Not Imported – Another common issue is that images may not import correctly to your new site. To fix this, you can try importing them as external images.
3. Redirects Not Working – If users are not being redirected correctly to your new site, then you need to carefully review your redirect settings. Make sure that your single install and the child site are both using the same Permalinks structure.
For other issues, see other common WordPress errors and how to fix them.
We hope this article helped you move a site from a WordPress multisite to a single install. You may also want to review our WordPress SEO checklist for your new install or try these essential WordPress plugins on your fresh site.
If you liked this article, then please subscribe to our YouTube Channel for WordPress video tutorials. You can also find us on Twitter and Facebook.
The post How to Move a Site from WordPress Multisite to Single Install first appeared on WPBeginner.
visual basic window form
how to create an app that employees can use to manage the main warehouse. The app must allow the user to enter the spare parts by serial number, name and/or use an external par code reader and specify which parts were dispatched to which aircraft notified by its number in the fleet. The app will then calculate the quantity of items for each type of spare part along with the price of the item then the App MUST give warnings about the remaining balance of the spare parts when it reaches only 4 items left in stock. If a user attempts to quarry about a certain item he should be able to do it using items serial number, or items name, an error message will be displayed informing the user that necessary input is missing. The GUI of the App must show the logo of the company (choose one of your own design) along with company name and contact details (address, telephone number, website... etc).
CSS Clogging
I recently visited a blog called "Does CSS loading cause blockage?" I opened it with a learning attitude, but it seemed that what was said was not completely correct, so I searched all kinds of information everywhere, and then I summarized it myself,
Will CSS Loading Cause Blockage?
First of all, the answer is definitely yes. The loading of css will not only block the parsing of HTML, but also block the execution of JS.
TextGears Adds Grammar Analysis to API Platform
TextGears provides an API platform for automated text analysis and correction, has now added a new Grammar Check API to its product offering. By adding this functionality, TextGears now provides developers with a one-stop shop for text analysis, grammar correction, and syntax moderation.
error with class, interface, or enum expected
help me to identify the problem with this code
public class Calculate
{
int service;
}
int price(int total, int amount, int currency)//overloading
{
int price(int total, int amount)//overloading
{
return service;
}
}
C:\Users\User\Documents\Calculate.java:12: error: class, interface, or enum expected
int price(int total, int amount, int currency)//overloading
^
C:\Users\User\Documents\Calculate.java:17: error: class, interface, or enum expected
}
^
this is the error:
IQVIA HealthCare Locator SDK Provides Detail on Millions of Healthcare Providers
IQVIA, a health information technology and clinical research solution provider, has announced a new HealthCare Locator SDK that provides developers with access to a healthcare information database that includes the details of 21.5 million healthcare providers and 2.5 million healthcare organizations globally.
to accept name,class and roll no and print them
please give me the whole program
can anyone help me please,its very urgent and my eyes is souring :(
Should DevTools teach the CSS cascade?
Stefan Judis, two days before I mouthed off about using (X, X, X, X) for talking about specificity, has a great blog post not only using that format, but advocating that browser DevTools should show us that value by selectors.
I think that the above additions could help to educate developers about CSS tremendously. The only downside I can think of is that additional information might overwhelm developers, but I would take that risk in favor of more people learning CSS properly.

I’d be for it. The crossed-off UI for the “losing” selectors is attempting to teach this, but without actually teaching it. I wouldn’t be that worried about the information being overwhelming. I think if they are considerate about the design, it can be done tastefully. DevTools is a very information-dense place anyway.
Direct Link to Article — Permalink
The post Should DevTools teach the CSS cascade? appeared first on CSS-Tricks.
You can support CSS-Tricks by being an MVP Supporter.