Promoting a local business can be challenging these days. Whether it’s because of oversaturation or complicated search engine algorithms, it’s all too easy to feel like no one will find your business in the local search results.
It’s even more frustrating when you offer great products and services, but customers just can’t seem to find you online.
![→ Download Now: SEO Starter Pack [Free Kit]](https://no-cache.hubspot.com/cta/default/53/1d7211ac-7b1b-4405-b940-54b8acedb26e.png)
Online local business directories and listing sites can change your situation and put your business front and center where your potential customers are already looking. As a local SEO specialist at my previous company, I know the ins and outs of how to get in front of your local target audience.
In this post, I’ll go over everything you need to know about creating business listings and why they’re so valuable, even in the age of social media and other marketing tactics.
Table of Contents
When you list your business in these directories, you build citations, a critical part of a local marketing strategy.
Today, Google is inserting itself between consumer and local business websites more often than ever before.
For proof, you need to look no further than Accelerated Mobile Pages (AMP), featured snippets, and, most importantly, local packs.
Local packs are the top or featured results Google displays when users make a location-based query.
The result typically shows three business listings with details such as the business name, customer reviews and ratings, physical location, opening and closing times, and phone number.
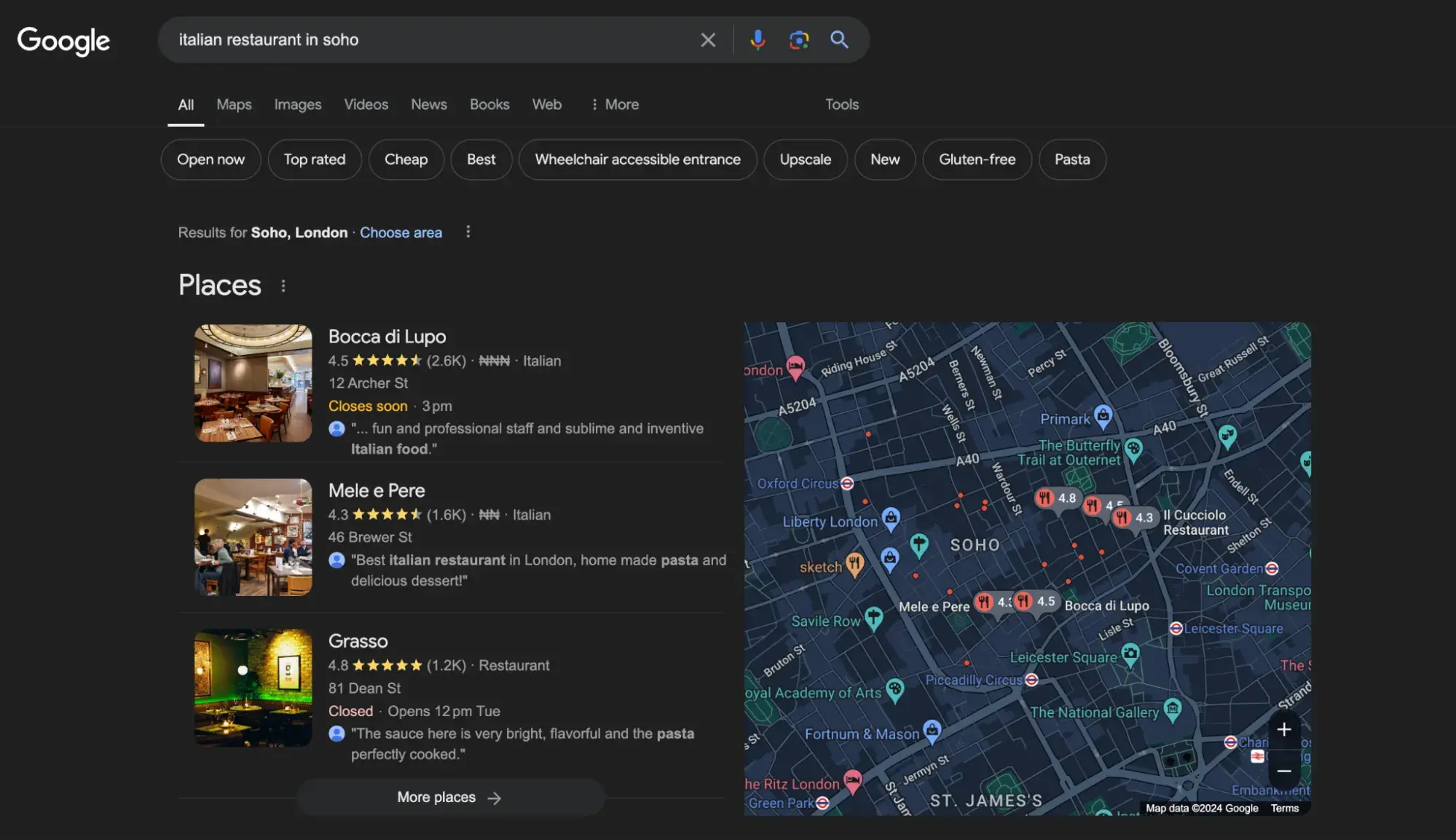
You can improve visibility in local packs by listing your local business' NAP (Name, Address, Phone Number) on directories, online business listing sites, and citation sites. Aside from improving your local SEO, these can also improve your rankings on search engines because the listings usually link back to your site.
But what is a business listing, and what does it look like?
When I was a local SEO specialist, I spent much of my time finding new business listing opportunities to build citations and generate backlinks for our local websites. These links allowed us to rank not only in the local packs but also in the main SERPs.
I have a few tips to help you make the most of your listing. Make sure your company listing has the following information once you add it to a directory:
- Consistent NAP. If you add or update your business on multiple business listing sites, ensure you provide the same company information across each directory. For instance, don’t use HubSpot in one listing and HubSpot Marketing in another.
- A link to your website. Backlinks or inbound links are crucial to your company website's domain rating. I recommend adding a tracking link at the end of URLs from directories to see how much traffic your website specifically gets from the business directories linking to it.
- A company description. Ensure you have a detailed description of your business that reflects your organization's mission, culture, and values. Such descriptions can help you attract the specific type of customers you serve.
- Multimedia. Give company searchers a visual taste of your business with a picture or video of your office, employees, or daily business operations.
While there are plenty of location-specific and industry-specific business listing sites, I recommend starting with bigger sites and working your way toward the more niche directories to promote your local business.
Before I share my list of the best local directories, let’s discuss the methodology I used to choose the sites.
How I Chose the Best Online Directories
I used to build citations for my former company, and I can confidently tell you that there are many, many business directories out there — and not all of them are good.
I used two simple parameters to curate the best business listing sites you can sign up for right now.
Domain Rating
Domain rating is a score given to websites to reflect how well they rank on Google based on their backlink profile. The minimum score is 0, and the maximum score is 100. Domain rating is similar to domain authority.
The minimum required domain rating for the directories on this list was 50. I gathered the data from Ahrefs (September 2024), but you can also double-check using your preferred SEO tool.
Category
Most of the directories on this list work for any industry or target market. However, I made some reservations for super popular directories that work for specific industries, such as healthcare and real estate.
I’ve included traffic numbers for your reference, but I don’t consider it the most important factor in choosing where to list your business. Any local citation helps you build consistent references to your NAP information online.
With that, let’s go over the top free business directories you can join right now.
The business listing sites below made the list because they are free and offer an easy process for claiming or creating a profile.
Some online business directories require phone verification or extra steps, but the options below are simple to sign up for. These ten business listings are a great place to start before signing up on more industry-specific listing sites.
1. Google Business Profile
Domain Rating: 92
Monthly Organic Traffic: 3.5B Visits
Google Business Profile (formerly Google My Business) lists you directly on Google’s local pack and search results. A Google Business Profile includes your NAP, business hours, services, customer reviews, photos, and more.
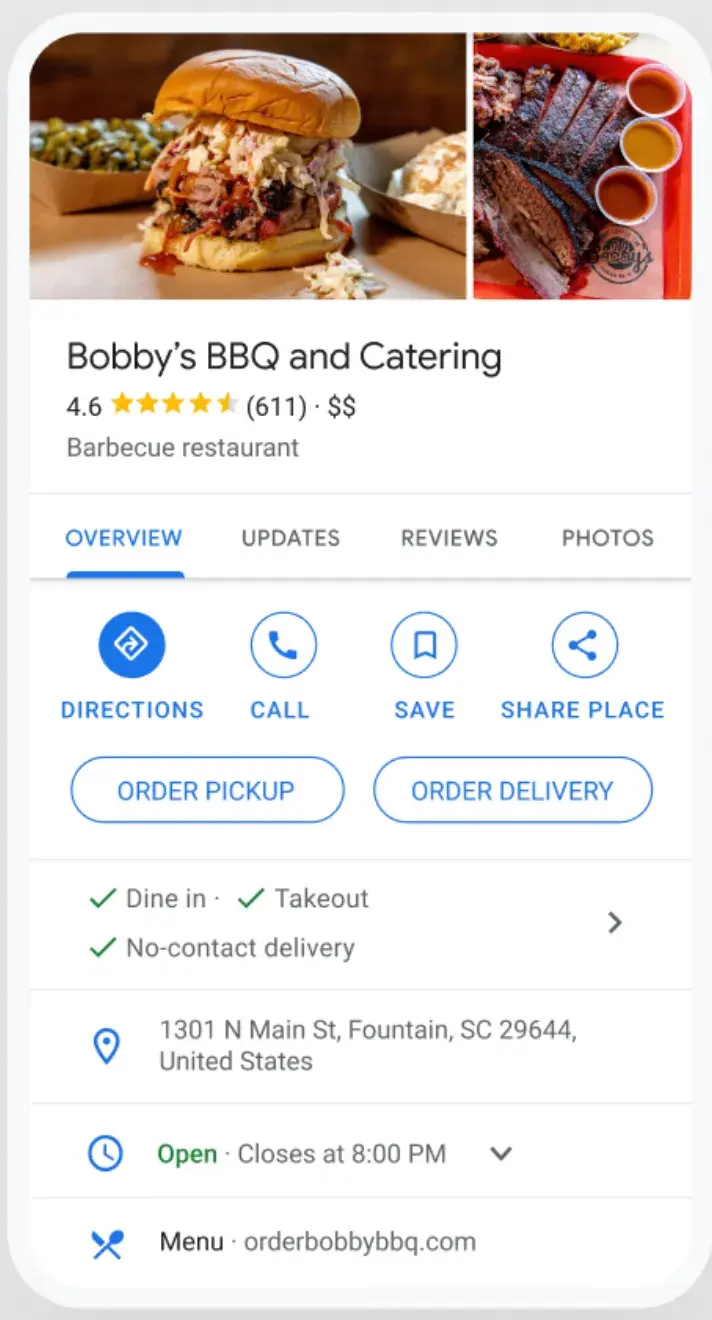
Claim your free Google Business Profile.
With over 22 billion daily Google searches, you’d be doing your business a great disservice if you don’t create a Google Business Profile.
2. Yellow Pages
Domain Rating: 90
Monthly Organic Traffic: 2.1M Visits
An oldie but a goodie, Yellow Pages is perhaps the most well-known local directory. A Yellow Pages listing includes your NAP, parking information, business hours, and customer reviews.
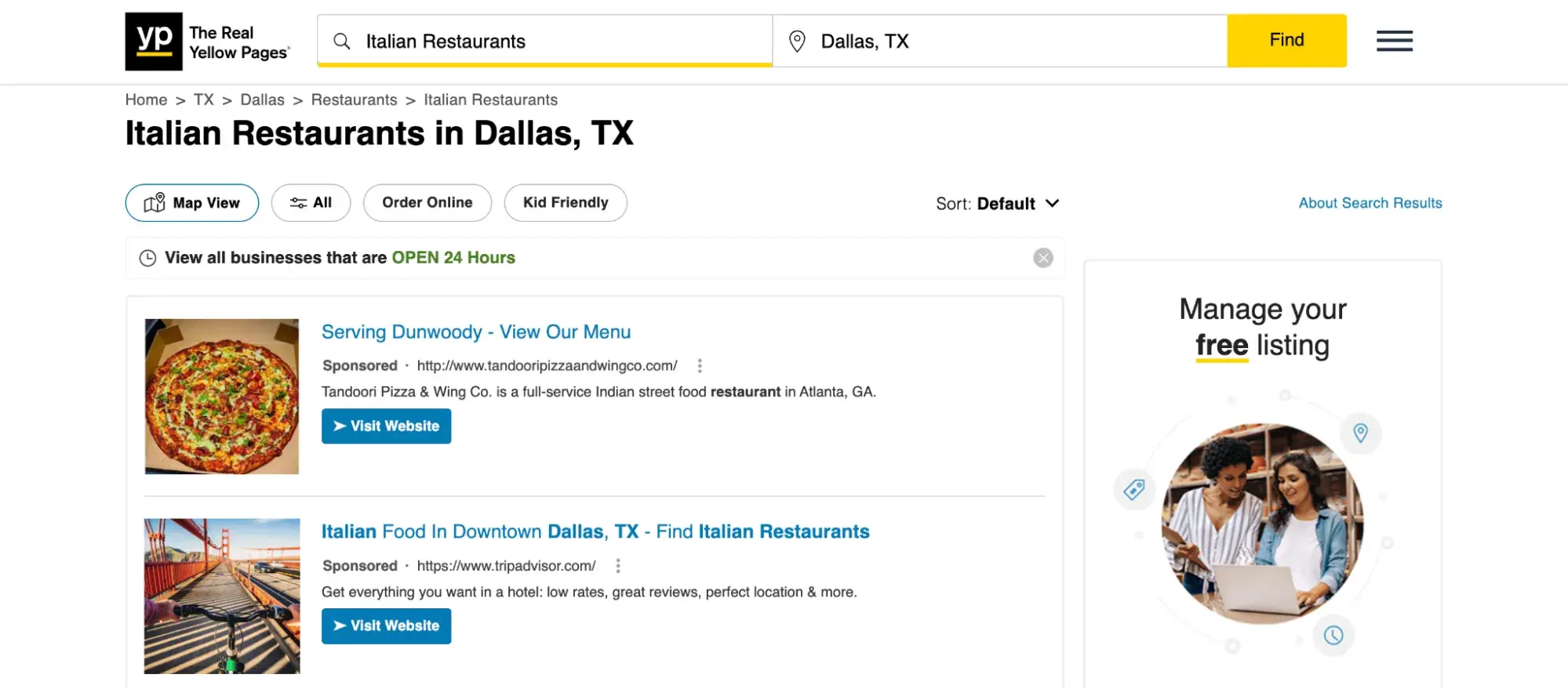
Claim your free business listing on Yellow Pages.
Given that Yellow Pages has been around for over 25 years, the site has built a reputation people trust.
3. Foursquare
Domain Rating: 91
Monthly Organic Traffic: 6.2M Visits
Foursquare is a general online directory for a wide variety of local business categories. A Foursquare listing includes your NAP, business hours, logo, and customer reviews.
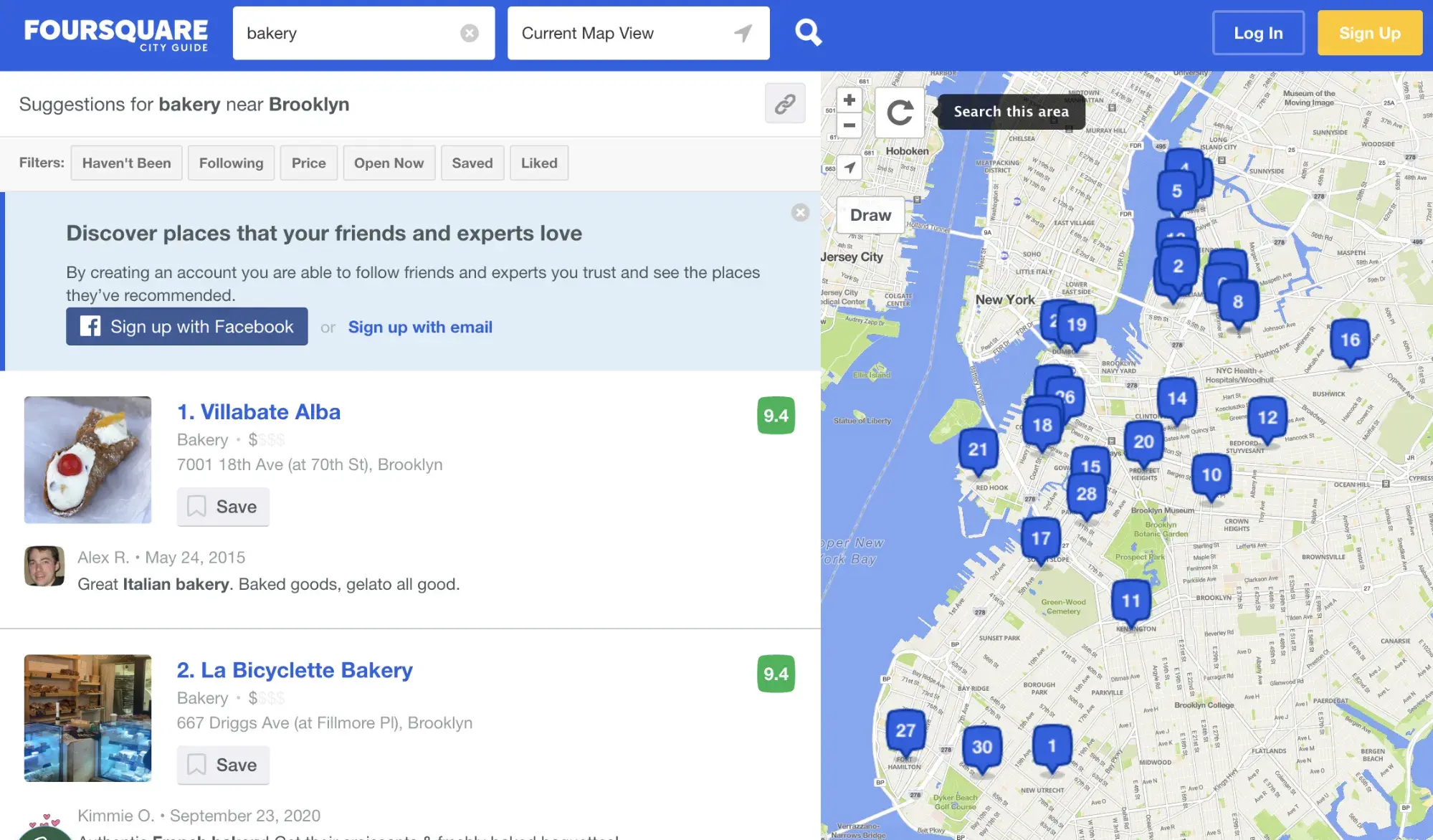
Claim your free business listing on Foursquare.
When building citations at my previous company, Foursquare was one of my favorite business listing sites. It’s quick, easy, and painless to add your online listing, and it includes all essential information without many frills.
4. Better Business Bureau
Domain Rating: 93
Monthly Organic Traffic: 7.2M Visits
Better Business Bureau is an online directory site for a wide variety of local business categories. A Better Business Bureau listing includes your NAP, company history, email, and website. You may also apply for optional accreditation.
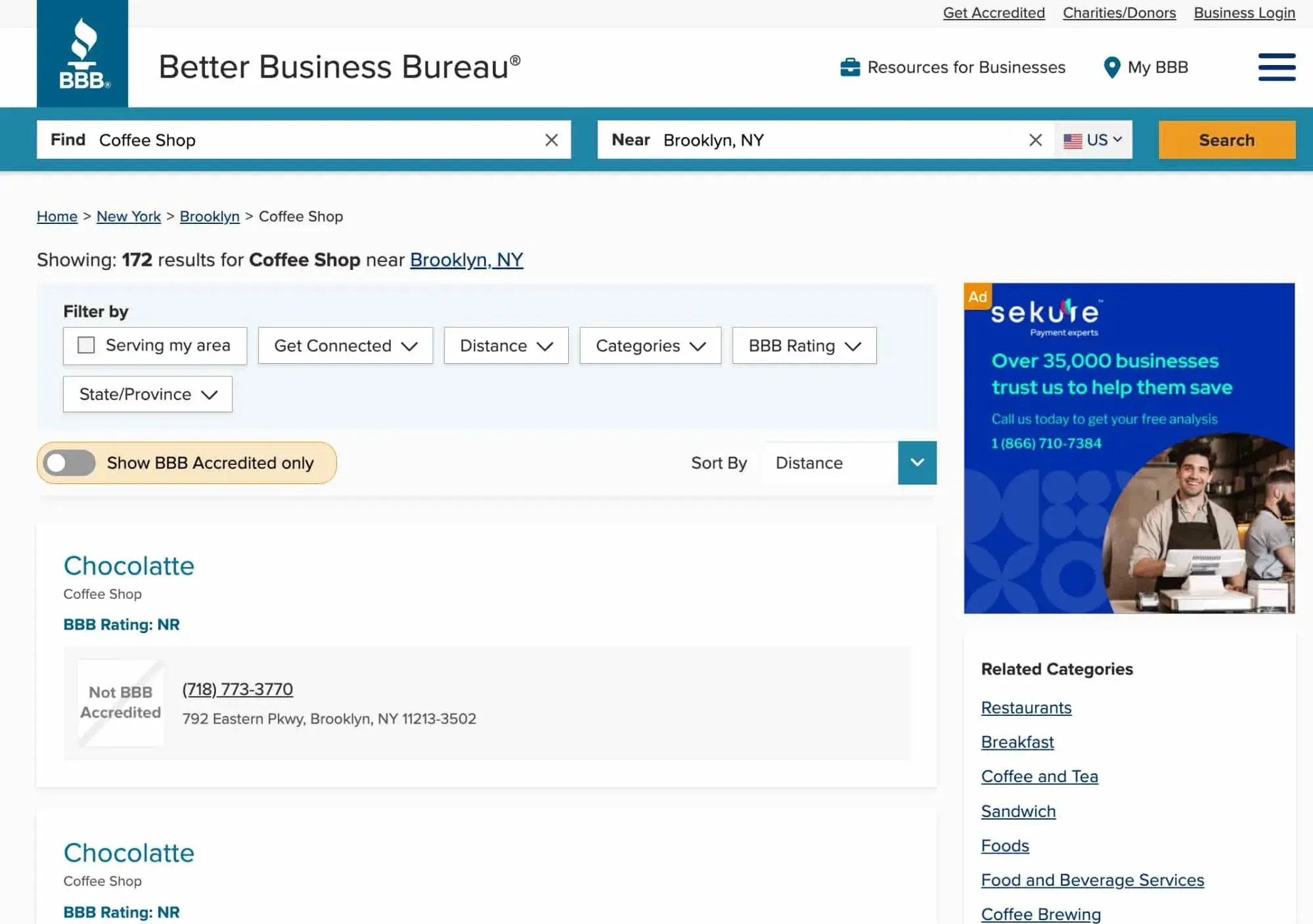
Claim your free business listing on BBB.
BBB accreditations add credibility to your listings and help you attract more customers. I also like that you can manage customer reviews and resolve complaints on BBB.
5. Superpages
Domain Rating: 85
Monthly Organic Traffic: 60K Visits
Superpages is a general online directory site for a wide variety of local business categories. A Superpages listing includes your NAP, business hours, and website.
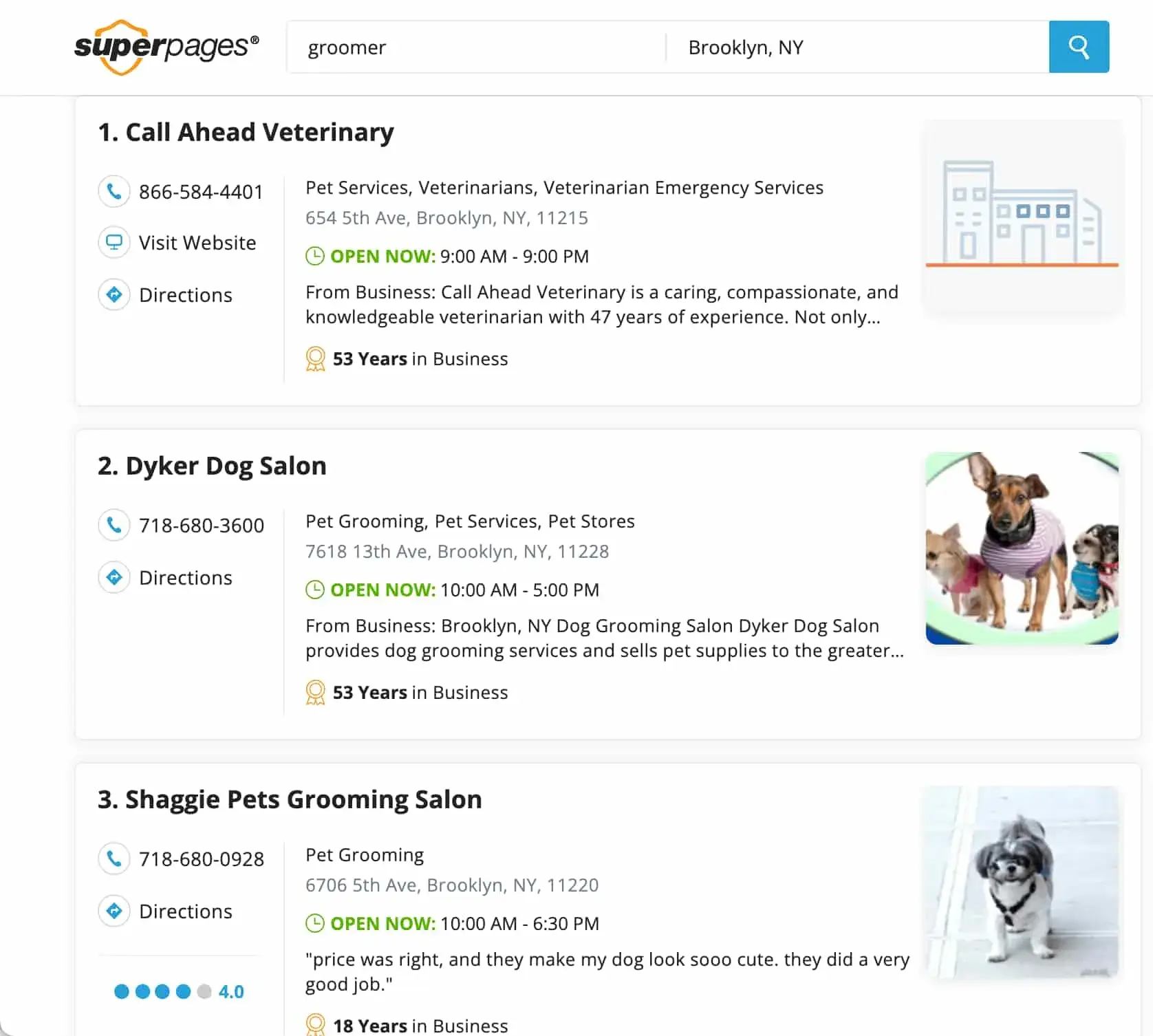
Claim your free business listing on Superpages.
The listings here are super detailed. You can pay for targeted ads to have your listing reach the right people at the right time.
6. Hotfrog
Domain Rating: 80
Monthly Organic Traffic: 6.2K Visits
Hotfrog is a general online directory site. A Hotfrog listing includes your NAP, business hours, business description, and website.
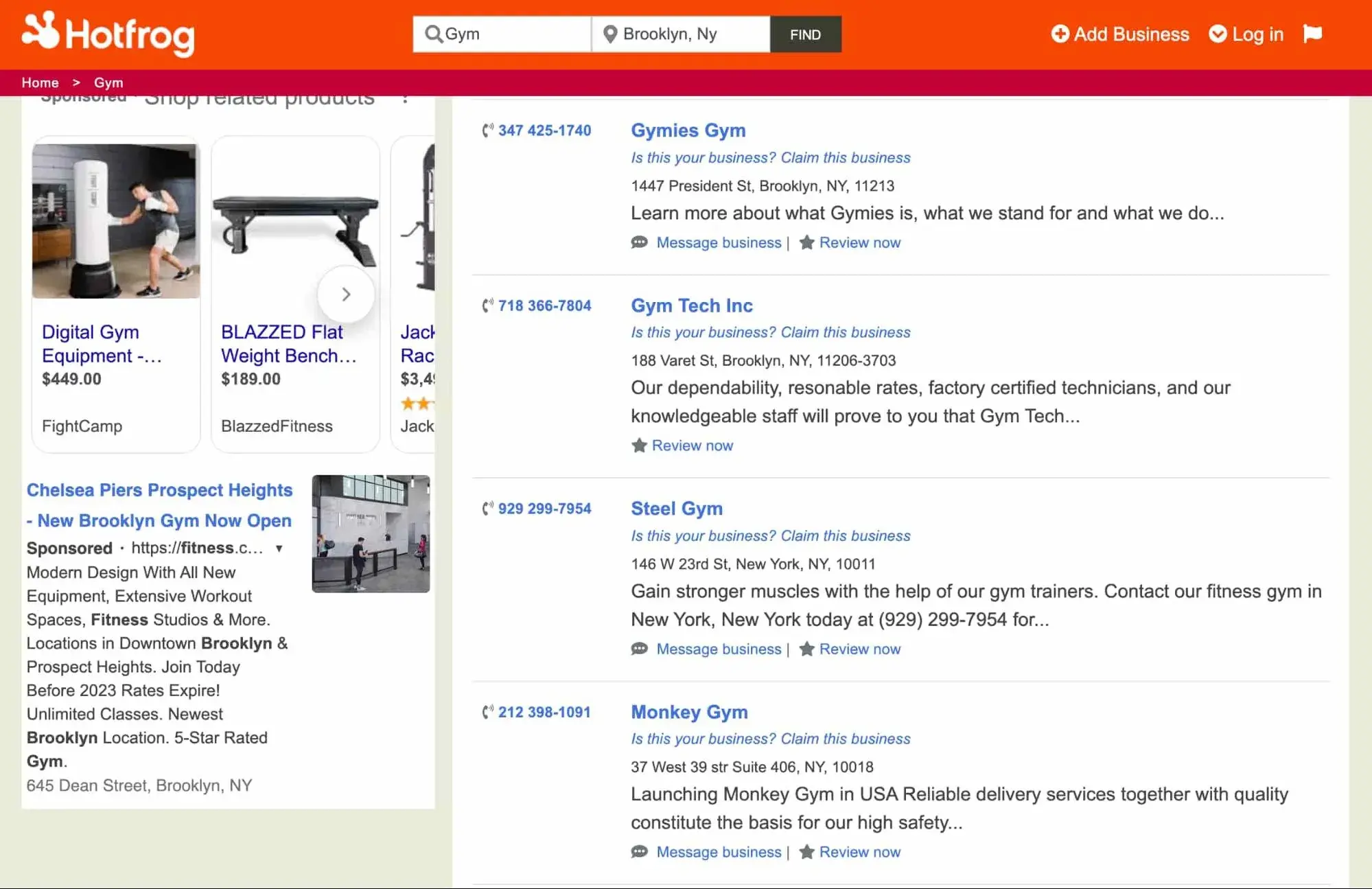
Claim your free business listing on Hotfrog.
Hotfrog’s user interface is simple, so users can quickly find the businesses they’re looking for. You also get valuable insights from Hotfrog about how users find your business.
7. Merchant Circle
Domain Rating: 85
Monthly Organic Traffic: 12.6K Visits
Merchant Circle is a general online listing site. A Merchant Circle listing includes your NAP, logo, payment options, business hours, business description, and website.
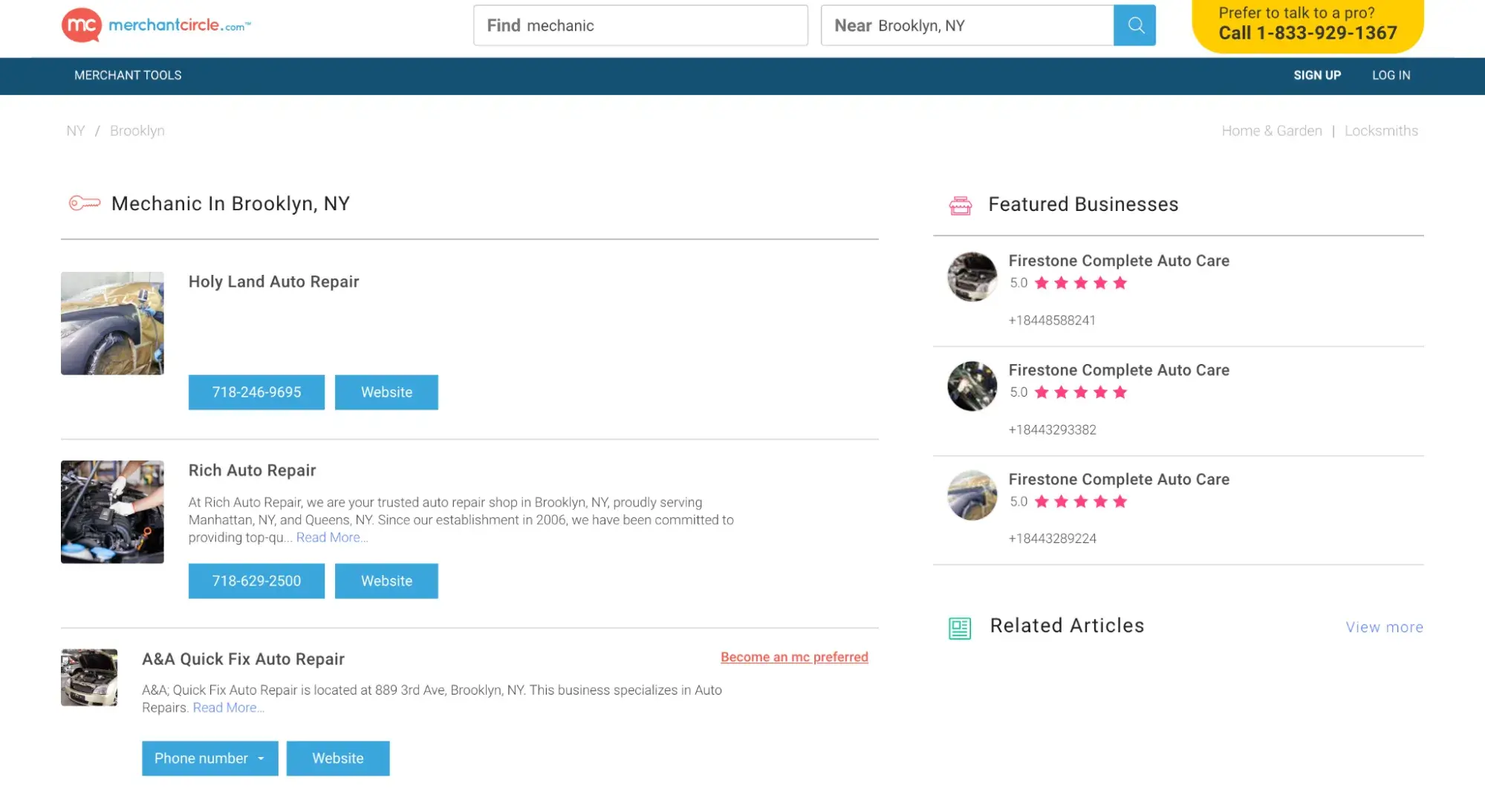
Claim your free business listing on MerchantCircle.
What’s great about Merchant Circle is that you can network with other business owners with listings on the site.
8. EZLocal
Domain Rating: 80
Monthly Organic Traffic: 8.6K Visits
EZLocal is a general online directory for a wide variety of local business categories. An EZLocal listing includes your NAP, parking information, business hours, and website.
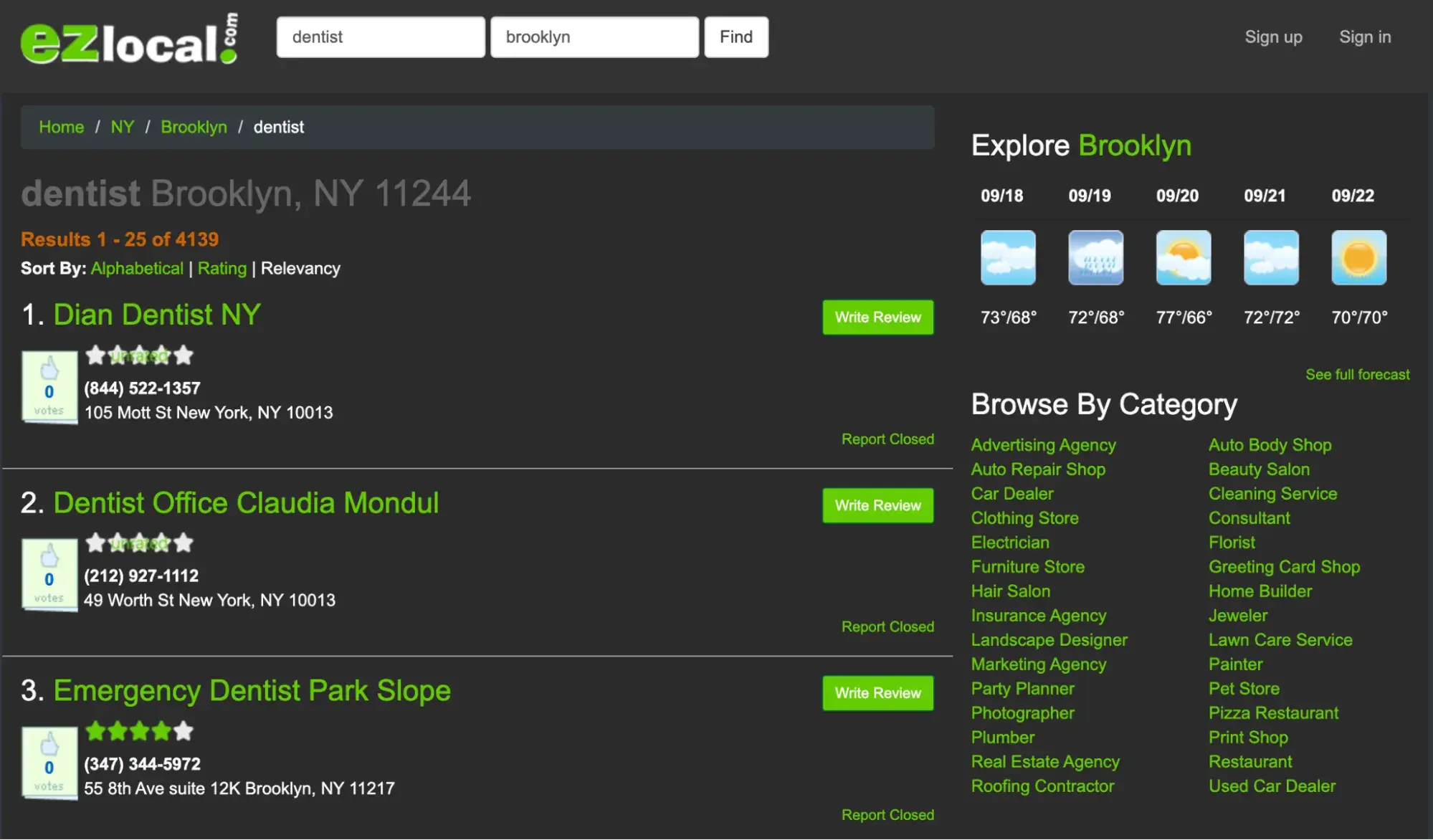
Claim your free business listing on EZLocal.
Business listings on EZLocal are often synchronized with other directories, thus improving your visibility on search engines like Google and Bing.
9. eLocal
Domain Rating: 77
Monthly Organic Traffic: 20.1K Visits
eLocal is a general local directory. An eLocal listing includes your NAP, business description, website, and payment options.
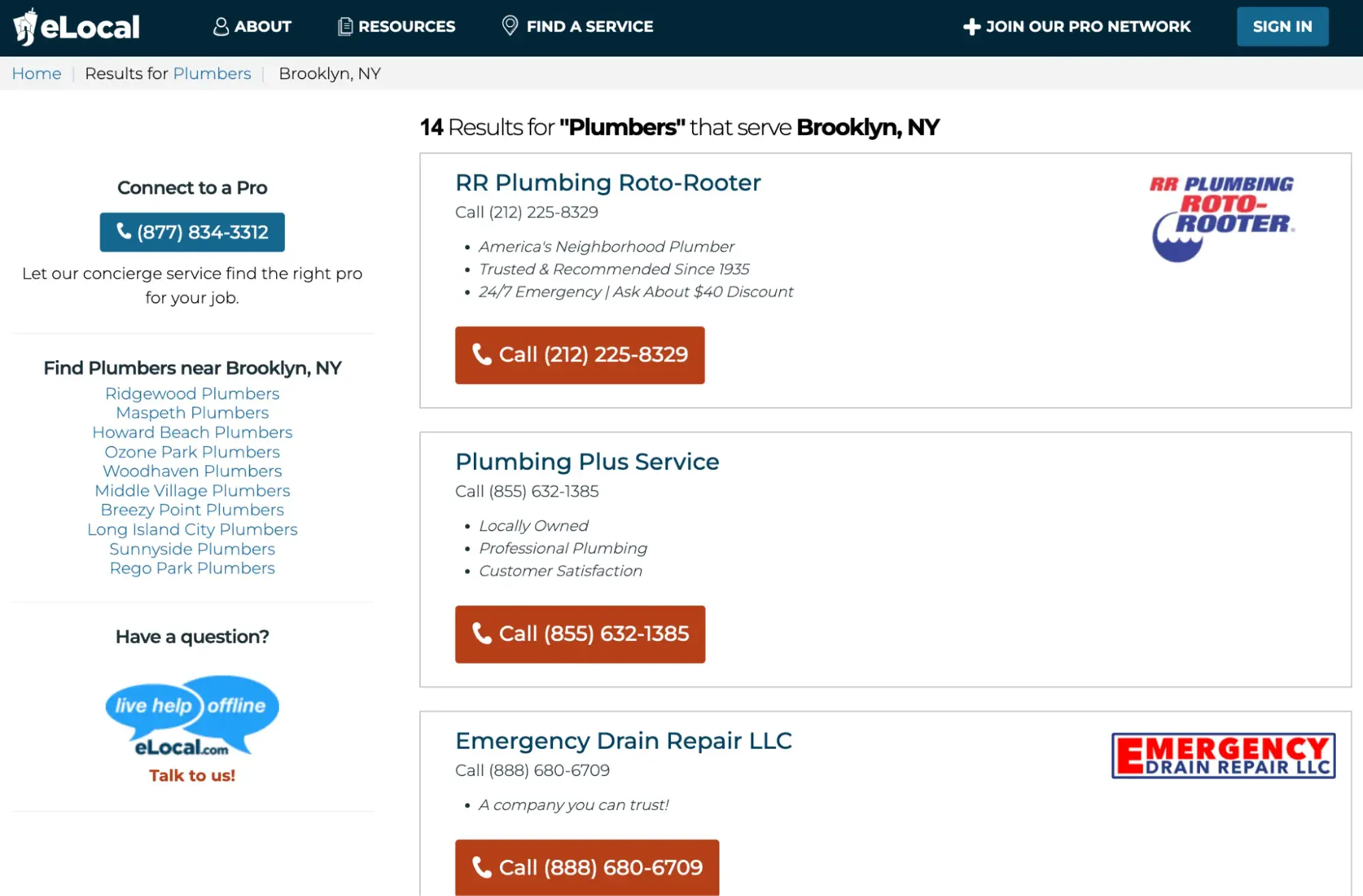
Claim your free business listing on eLocal.
In addition to free listings, you can pay to have eLocal boost your business to potential customers.
10. Manta
Domain Rating: 87
Monthly Organic Traffic: 412K Visits
A Manta listing includes your NAP, business hours, services, website, business description, and social media links. You may also apply for Manta verification.
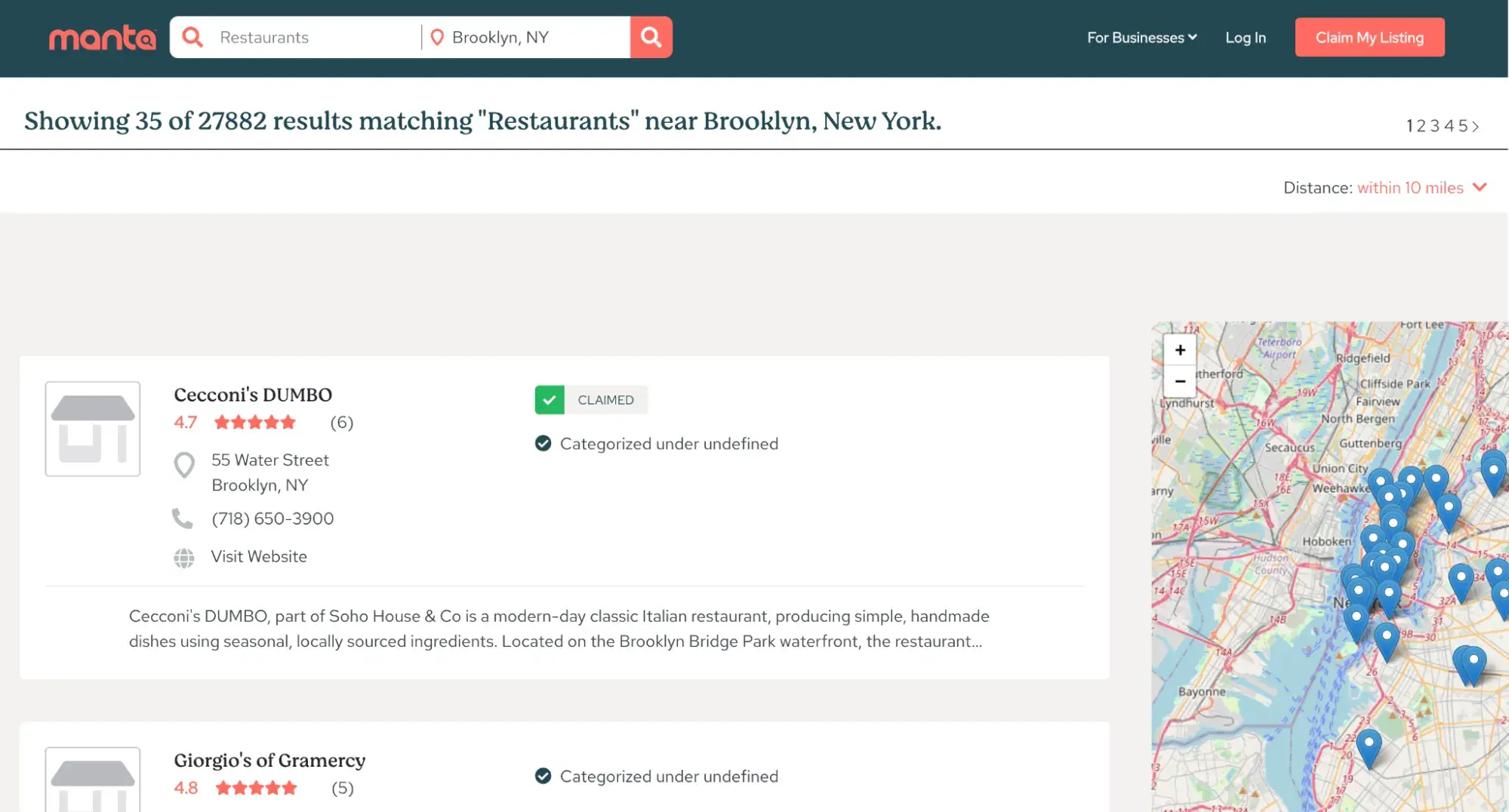
Claim your free business listing on Manta.
Aside from listing websites, Manta has an all-in-one business management software to help small businesses grow online.
Best Online Directories for Local Businesses
When listing your business online, you aren‘t limited to using the free sites above.
I’ve compiled the best directories on the web for listing your business, whether paid or unpaid (most, however, should be free).
In my years as a local SEO and citation builder, I found most of these relatively easy to sign up on, but a few sites may require additional verification or a pending period. The good news is that there is little to no chance that these sites will reject your listing.
1. Facebook Pages
Domain Rating: 100
Monthly Organic Traffic: 1.7B (Facebook.com)
Facebook Pages is one of the best places to list your business online and get visibility through both organic search and Facebook’s internal search feature.
A Facebook Page includes both basic NAP information and updates from your business. With 31% of people preferring to use social media for search, you don’t want to neglect your presence there!
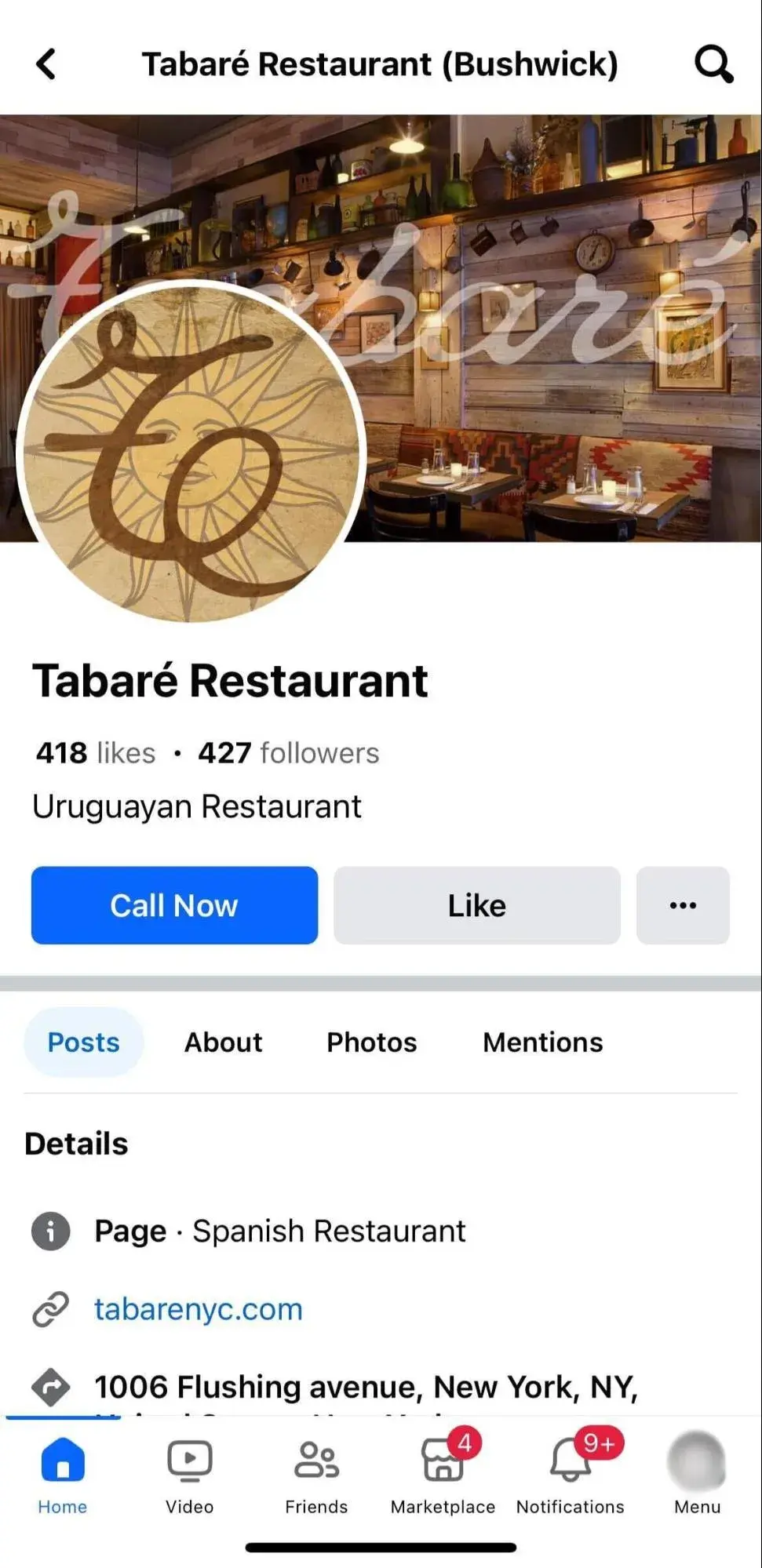
Create your free business listing on Facebook Pages.
2. Instagram for Business
Domain Rating: 99
Monthly Organic Traffic: 1.6B (Instagram.com)
Like Facebook, Instagram is another valuable place to list your business’ NAP information for visibility, local SEO, and social media marketing. An Instagram profile will include your business’ NAP information, photos, and updates that you post directly.
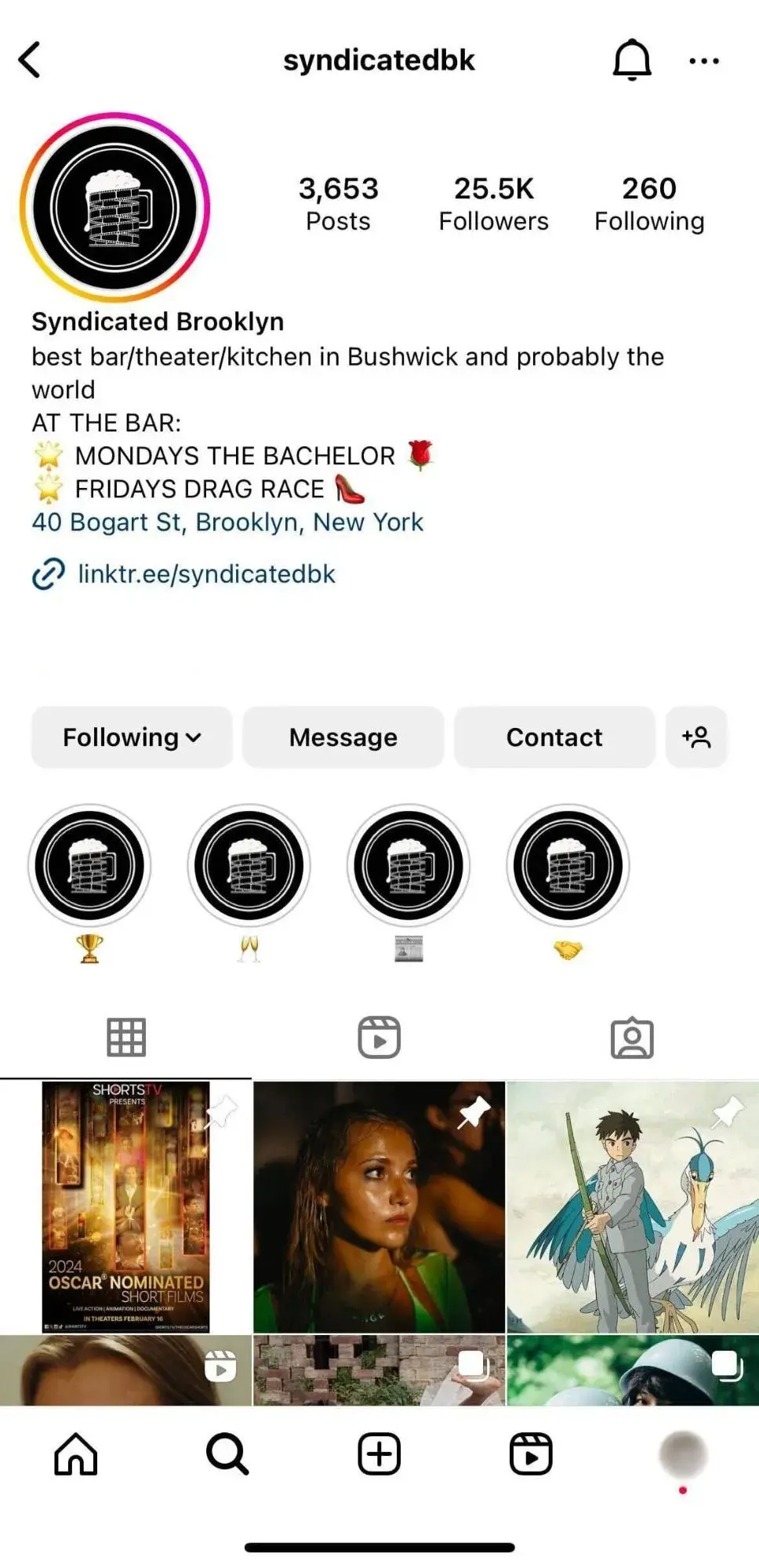
Create your free business listing on Instagram.
3. LinkedIn Company Directory
Domain Rating: 98
Monthly Organic Traffic: 7.1K
LinkedIn offers a directory of business pages (called LinkedIn Pages), where most companies and businesses can add a profile for free. Like Facebook and Instagram, you can list your NAP and publish updates and jobs.
Companies are listed alphabetically on the Company Directory landing page, and users can find your business by navigating to “Browse By” and selecting the first letter of your business name.
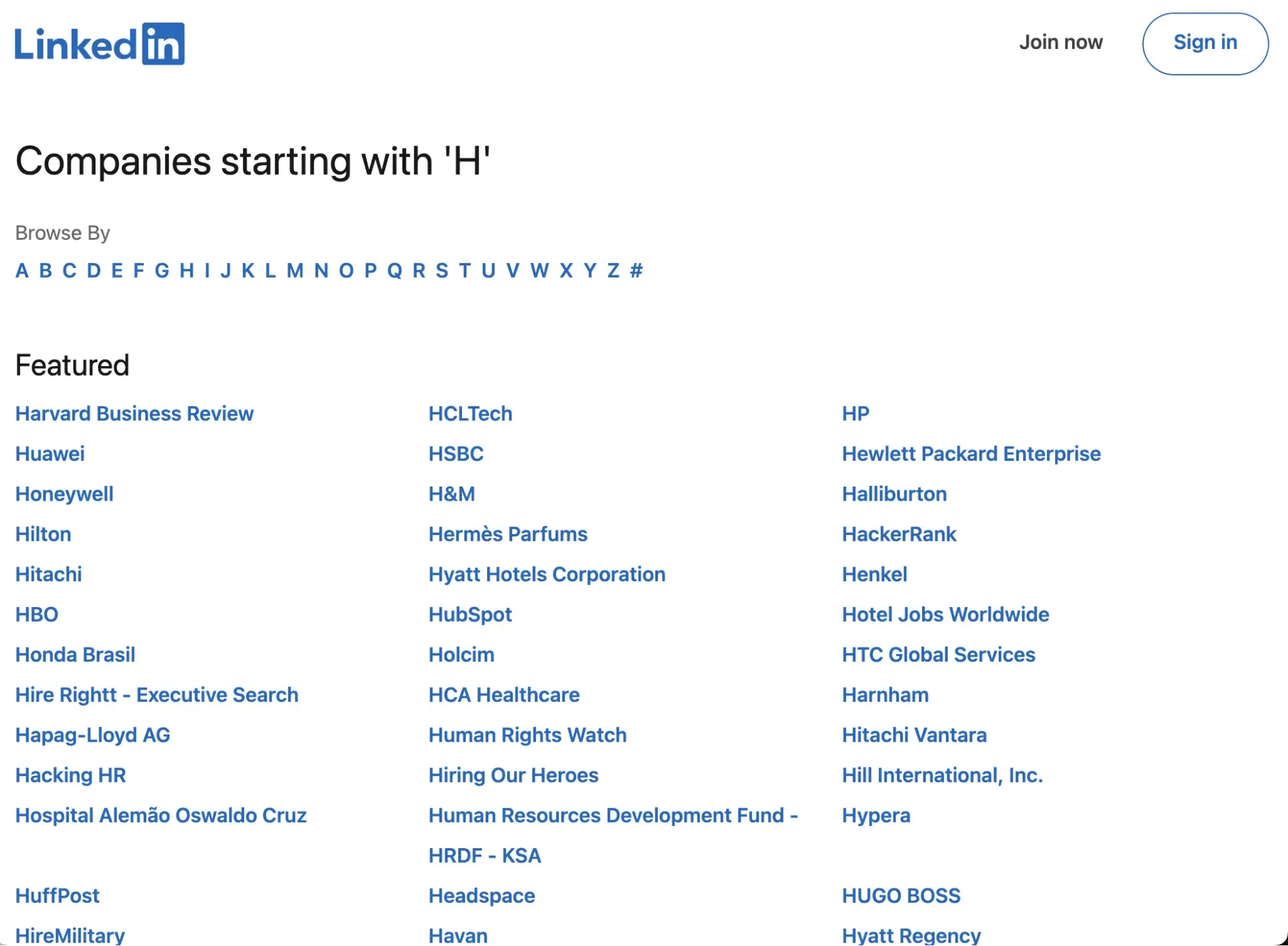
Create your free business listing on LinkedIn.
You can also post directly from your LinkedIn business account and get found by over 1 billion LinkedIn users.
4. Apple Maps
Domain Rating: 97
Monthly Organic Traffic: 125K
If people visit your business in person, adding it to navigation apps and services is always a good idea. Apple Maps isn’t technically a business listing site, but it’s still an essential citation. You can now easily get started by using Apple’s new Business Connect portal.
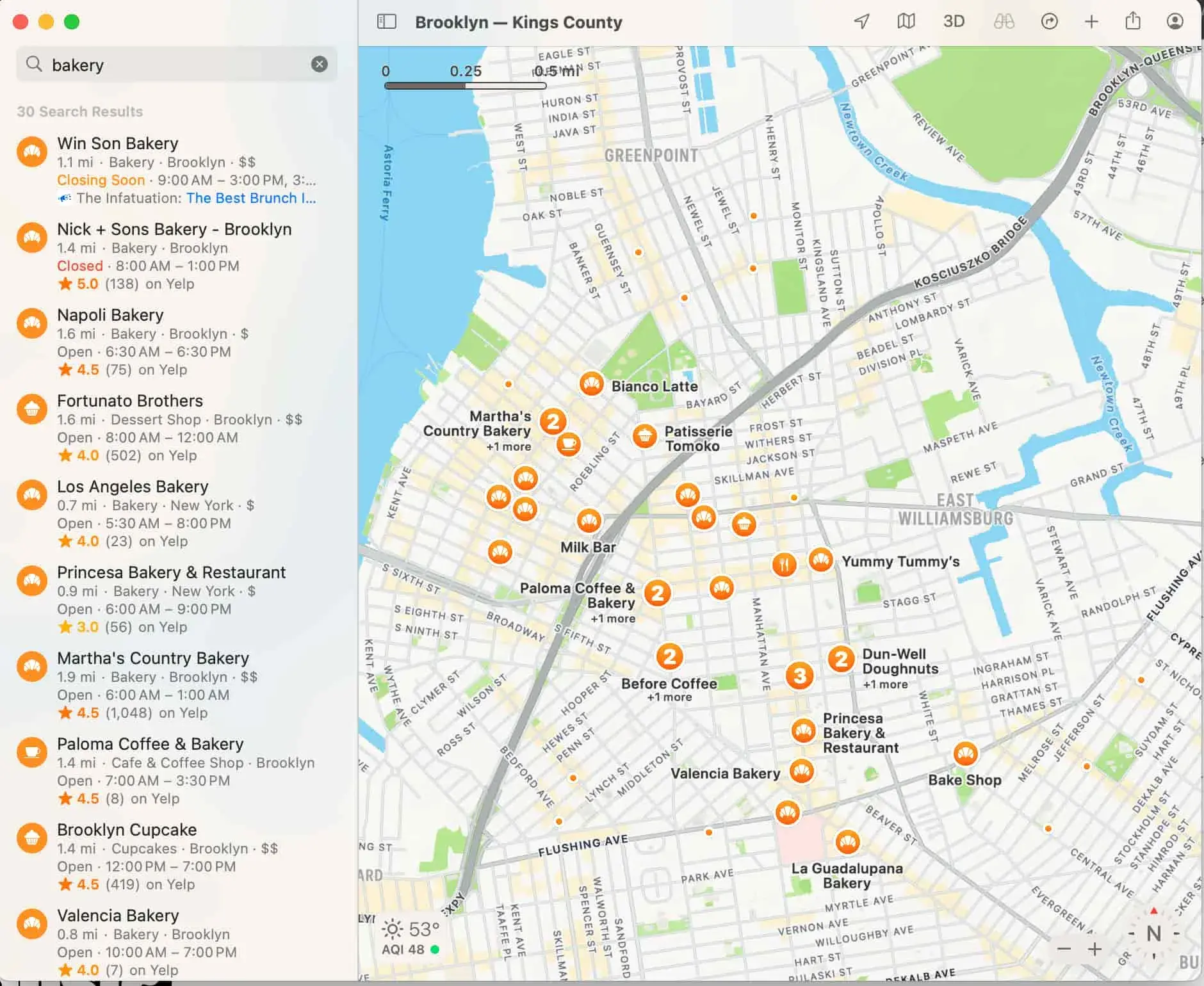
Create your free business listing on Apple Maps.
5. Yelp
Domain Rating: 95
Monthly Organic Traffic: 98M
Whether you run a restaurant, a local boutique, or a repair shop, Yelp is an invaluable directory to list your business online. It boosts your credibility with customer reviews and provides one more citation with your NAP, business hours, services, and more.
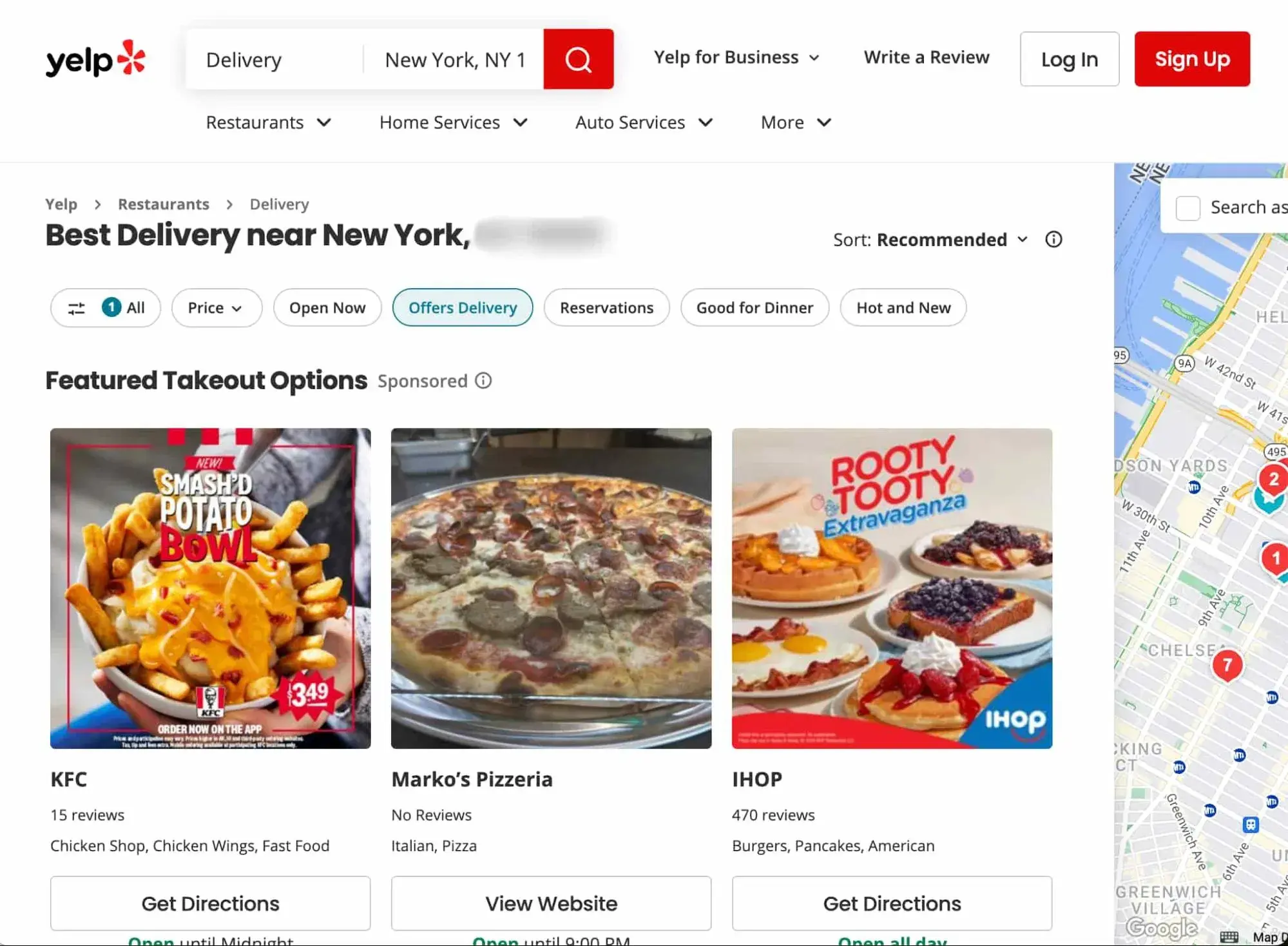
Create your free business listing on Yelp.
6. Bing Places
Domain Rating: 82
Monthly Organic Traffic: 413K
Bing may not feel as essential as Google, but it’s still an important citation, especially because some of your customers might use Bing as their primary search engine. For instance, in China, where Google is banned, Bing is the primary search engine.
Adding your business to Bing also allows users to navigate directly to you using Bing Maps.
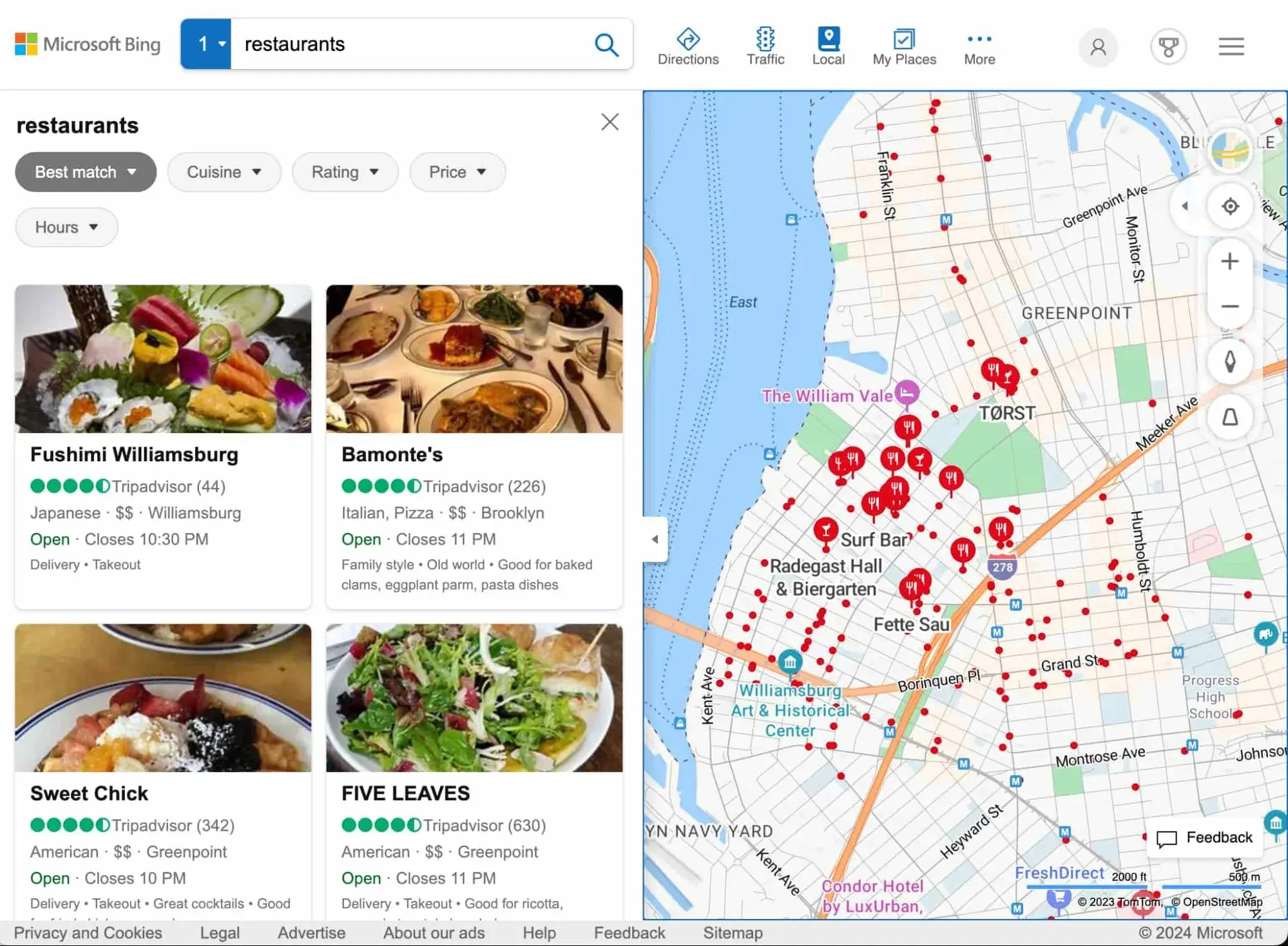
Create your free business listing on Bing Places.
7. Angi
Domain Rating: 90
Monthly Organic Traffic: 4.3M
Formerly known as Angie’s List, Angi is a valuable citation for home services — from plumbing to landscaping to maintenance and renovations.
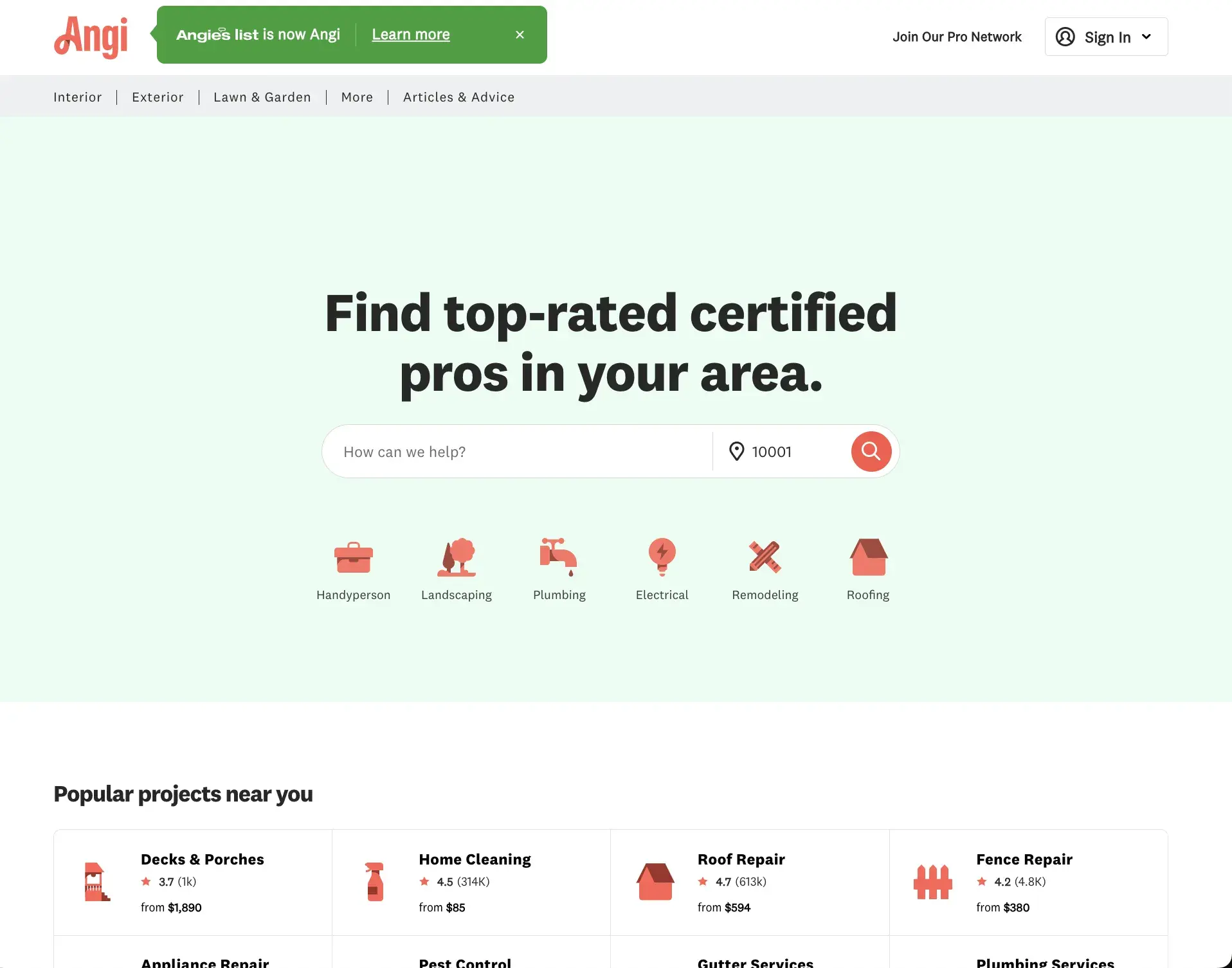
Create your free business listing on Angi.
8. Thumbtack
Domain Rating: 90
Monthly Organic Traffic: 1M
Thumbtack is another excellent business directory for providers of home renovation and maintenance services. Other industries that could add a business listing to Thumbtack include electronics repair specialists and web designers.
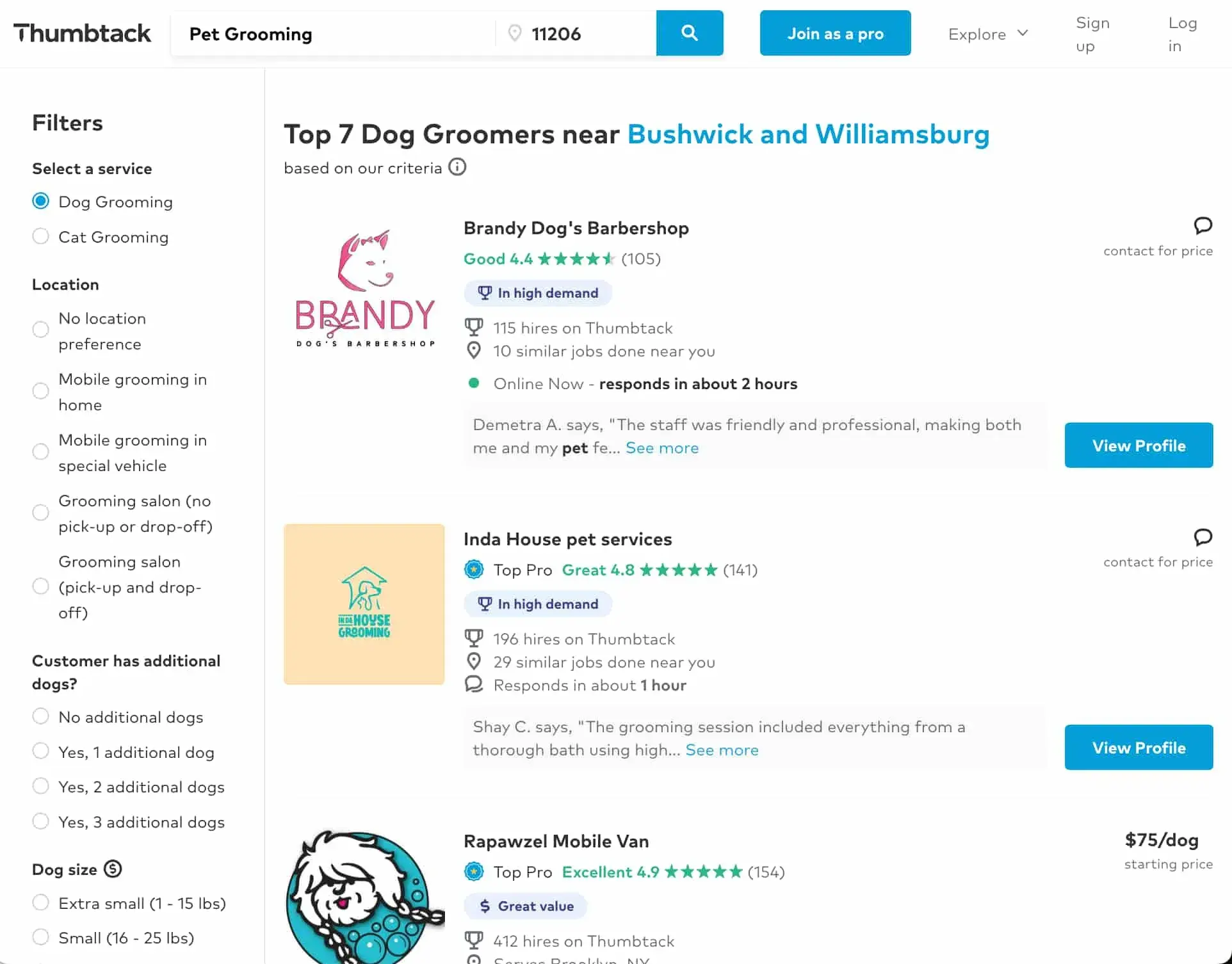
Create your free business listing on Thumbtack.
9. Nextdoor
Domain Rating: 90
Monthly Organic Traffic: 3.8M
Nextdoor is a neighborhood guide and a free online business directory for local businesses of any category. I especially recommend it if you offer home services or products at a local shop.
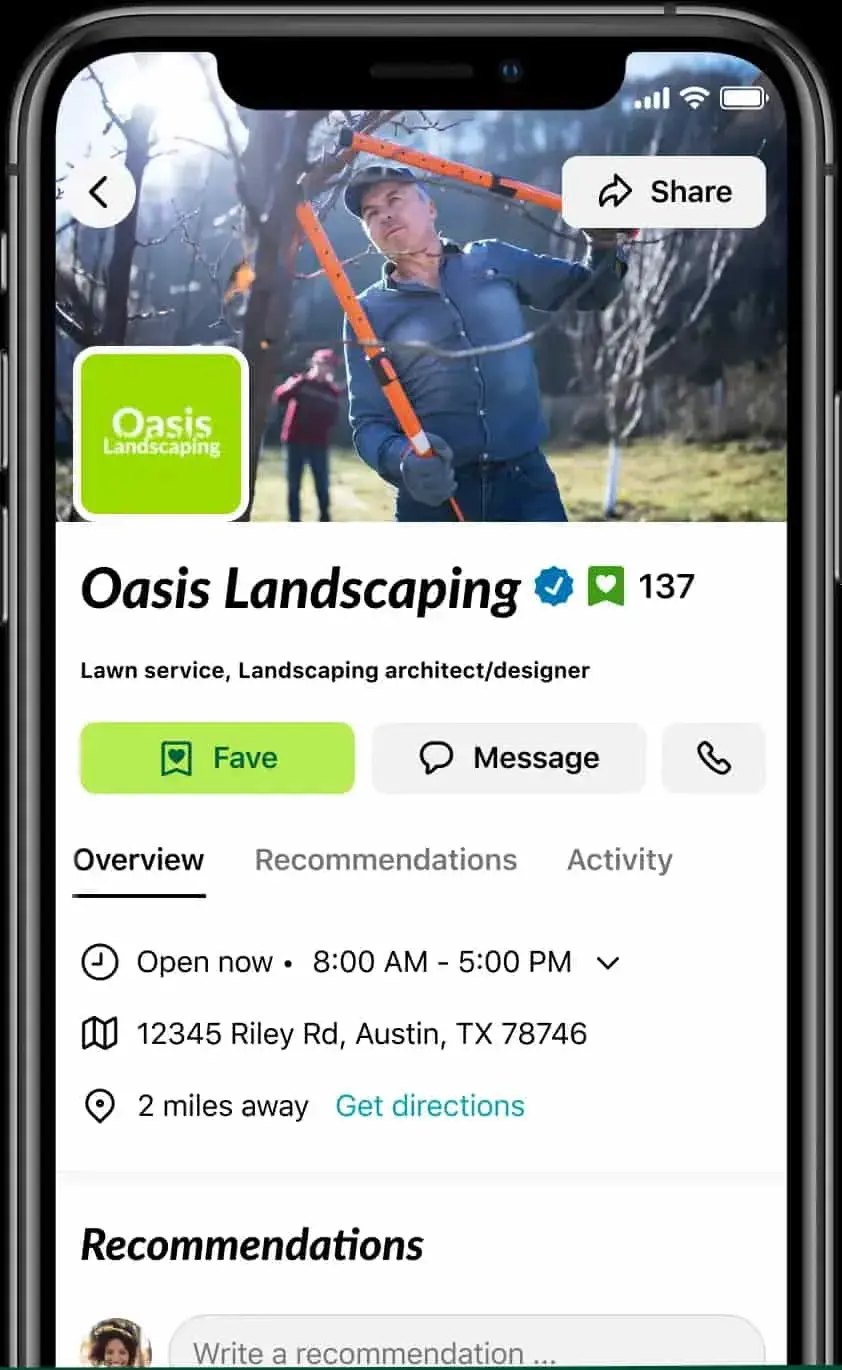
Create your free business listing on Nextdoor.
10. Zocdoc
Domain Rating: 88
Monthly Organic Traffic: 2.1M
Zocdoc is an online directory that makes it easy to book appointments with healthcare specialists. I recommend it if you provide healthcare services.
You can set your availability on the website and receive and manage client reviews. The only drawback is that this site is only available in the United States.
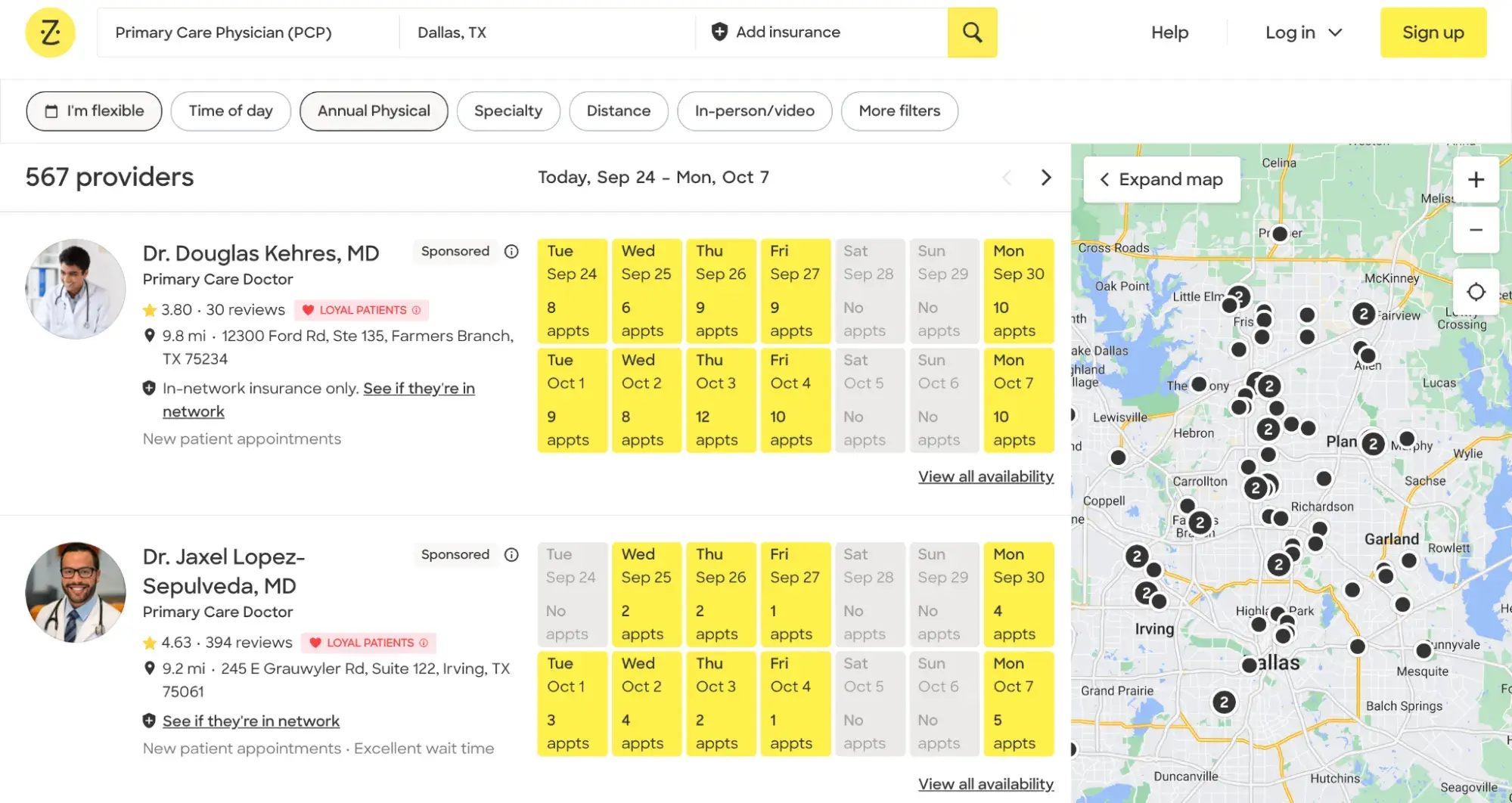
Create your business listing on Zocdoc.
Best Business Listing Sites
Those aren’t all the online directories you can join. Below are some of the best options for businesses of any category.
You’ll notice that some of them have low organic traffic numbers. Remember: The main benefit of adding your company to business listing sites is to build citations with your NAP information.
Even if an online directory only receives a few organic visits per month, that’s okay — the main value is having a listing with your correct name, address, and phone number (NAP).
Don’t worry about upgrading any of these listings. I never did when I was building citations. Most people no longer visit online business directories; instead, they use Google or other popular websites like Yelp.
1. HubSpot Solutions Online Directory
Domain Rating: 93
Monthly Organic Traffic: 100 Visits
HubSpot offers an online directory for marketing and advertising agencies. If you fall into this category, I highly recommend signing up for free.
Add your free business listing to HubSpot’s Solutions directory.
While the traffic numbers here are low, people who visit this directory know precisely what they’re looking for.
2. City-Data
Domain Rating: 84
Monthly Organic Traffic: 730K
City-Data is a neighborhood forum and online directory covering the entire U.S. I like how City-Data provides demographic and statistical data. Here, you can find stats like median household income and unemployment percentages.
Create your free business listing on City-data.com.
3. Chamber of Commerce
Domain Rating: 82
Monthly Organic Traffic: 23.8K
Chamber of Commerce is an online directory with a membership and an accreditation option. Membership on the Chamber of Commerce website adds credibility to your business and makes you more attractive to potential customers.
Create your free business listing on ChamberofCommerce.com.
4. Brownbook.net
Domain Rating: 79
Monthly Organic Traffic: 2.6K
Brownbook.net is a Yellow Pages alternative for businesses of any category. It's one of my favorite citations, and it’s very easy to sign up.
With a presence in over 200 countries, a Brownbook listing opens your business to global exposure.
Create your free business listing on Brownbook.net.
5. Sales Spider
Domain Rating: 70
Monthly Organic Traffic: 617
Sales Spider is an online business directory with over 80 million global businesses. The site’s filtering capabilities allow users to quickly connect with businesses offering the services they want.
Create your free business listing on Sales Spider.
6. Kompass.com
Domain Rating: 77
Monthly Organic Traffic: 844K
Kompass is a B2B online business directory that also allows you to respond to public proposal requests. Kompass supports multiple languages, allowing businesses to penetrate international markets.
Create your business listing on Kompass.com.
7. Storeboard
Domain Rating: 77
Monthly Organic Traffic: 3.2K
Storeboard is an online business directory with a homepage “feed,” like a social media site. This social media element makes it easy for users to find your business and can potentially increase your reach.
Create your free business listing on Storeboard.
8. eBusinessPages
Domain Rating: 77
Monthly Organic Traffic: 231
eBusinessPages may have low traffic numbers, but it’s an easy and simple citation to add to your roster.
Create your free business listing on eBusinessPages.
9. City Squares
Domain Rating: 74
Monthly Organic Traffic: 27K
CitySquares is an online business directory with a handy partner program for companies with multiple locations. You can easily customize your listing and integrate multiple payment methods.
Create your free business listing on City Squares.
10. BOTW
Domain Rating: 74
Monthly Organic Traffic: 8.1K
Best of the Web (BOTW) is an online business directory with advertising and upgrade options. BOTW analytics can help you optimize your listing and attract more customers.
Create your free business listing on BOTW.
11. Infobel
Domain Rating: 74
Monthly Organic Traffic: 148K
Infobel is an EU-based business listing site with a local U.S. directory for companies of any category.
Create your free business listing on Infobel.
12. iBegin
Domain Rating: 73
Monthly Organic Traffic: 4.9K
iBegin is a simple online directory where you can submit your business and blog posts. Listing your site here increases your chances of appearing in relevant search queries in your area.
Create your free business listing on iBegin.
13. Neustar Localeze
Domain Rating: 73
Monthly Organic Traffic: 69K
Neustar Localeze is a business listing service that also includes an online directory. This one is paid, and sign-up can be a little more complicated than other options, but it’s still worth it, in my opinion.
Create your business listing on Neustar Localeze.
14. GoLocal247
Domain Rating: 73
Monthly Organic Traffic: 600
GoLocal247 is an online business directory where you can also post free classified ads. You can easily customize your listing on GoLocal247 so users always get the most accurate information about your business.
Create your free business listing on GoLocal247.
15. Cybo
Domain Rating: 74
Monthly Organic Traffic: 660K
Cybo is a global business listing site for companies of any category. You get to improve customer engagement as Cybo allows you to interact with customers right on the website.
Create your free business listing on Cybo.
16. Just Landed
Domain Rating: 71
Monthly Organic Traffic: 5.9K
Just Landed is an online business directory that includes an expat community and an area for classified ads. If you own a business that caters to this community, you should definitely get listed on Just Landed.
Create your free business listing on Just Landed.
17. Yellow.Place
Domain Rating: 73
Monthly Organic Traffic: 30K
Yellow.Place is a Yellow Pages alternative with advertising options. It focuses on the accuracy of business listings, which makes its verification process a bit more lengthy than other business directories.
Create your free business listing on Yellow.Place.
18. Hub.biz
Domain Rating: 72
Monthly Organic Traffic: 133K
Hub.biz is an online directory with a text-based “feed” that includes updates from local businesses.
Create your free business listing on Hub.biz.
19. Dun & Bradstreet Business Directory
Domain Rating: 79
Monthly Organic Traffic: 6.9K
Dun & Bradstreet (D&B) is a famous online directory with an accreditation option. D&B does more than connect you with potential customers. You can also expand your business opportunities by networking with possible partners and investors.
Create your free business listing on Dun & Bradstreet.
20. Turbify
Domain Rating: 86
Monthly Organic Traffic: 11.8K
Turbify is an online local listing provider that gives you a free report and lists you online. It also offers other tools and services, like a website builder and domain name registration, that help small businesses thrive.
Create your free business listing on Turbify.
21. n49
Domain Rating: 74
Monthly Organic Traffic: 3.5K
n49 is a Canada-based directory with a U.S. arm. It’s simple to sign up, and editing your listing is easy through its portal.
Create your free business listing on n49.
22. Cylex US
Domain Rating: 75
Monthly Organic Traffic: 25K
Cylex US is an online directory that offers premium listings and the ability to post special offers. If your business gets plenty of searches, there’s a chance to get featured on Cylex’s homepage and gain even more traffic.
Create your free business listing on Cylex.us.
23. Fyple
Domain Rating: 68
Monthly Organic Traffic: 1.2K
Fyple is a local directory for businesses of any category. It also allows customers to post reviews.
Create your free business listing on Fyple.
24. Opendi
Domain Rating: 68
Monthly Organic Traffic: 6.8K
Opendi is a business directory with international arms in Europe and South America. Opendi has a long pending period for new listings, but I believe it’s worth submitting.
Create your free business listing on Opendi.us.
25. Show Me Local
Domain Rating: 79
Monthly Organic Traffic: 29.9K
Show Me Local helps businesses improve their local visibility through optimized listings. The site also has tools for running time-sensitive promotions and discounts.
Create your free business listing on Show Me Local.
26. My Huckleberry
Domain Rating: 64
Monthly Organic Traffic: 50
My Huckleberry is an online business directory with an online forum and a coupon marketplace.
Create your free business listing on My Huckleberry.
27. Bizhwy
Domain Rating: 67
Monthly Organic Traffic: 284
Bizhwy is a simple online business directory that also allows you to submit a press release. Since it has low traffic numbers, I recommend sticking to a free listing.
Create your free business listing on Bizhwy.
28. DirJournal
Domain Rating: 65
Monthly Organic Traffic: 221
DirJournal is an online business directory that also offers advertising options. Although DirJournal’s traffic is low, it has a thorough listing review process that ensures only credible listings.
Create your free business listing on Dirjournal.
29. USdirectory
Domain Rating: 59
Monthly Organic Traffic: 800
USdirectory.com is a business listing site that offers advertising options. Although it attracts slightly higher traffic than DirJournal and Bizhwy, I still recommend sticking to a free listing for this one.
Create your business listing on USdirectory.
30. FindUSlocal
Domain Rating: 61
Monthly Organic Traffic: 700
FindUSlocal is an online business directory with a feed-like homepage. In addition to listings, the site contains information about local news, concerts, and sports events.
Create your free business listing on FindUSLocal.
31. MapQuest
Domain Rating: 91
Monthly Organic Traffic: 20M
MapQuest has a long history as a reputable navigation tool. Like Google Maps and Apple Maps, MapQuest has features that make it easy for shoppers to discover local businesses.
Create your business listing on MapQuest.
32. PennySaver USA
Domain Rating: 57
Monthly Organic Traffic: 2.9K
PennySaver USA focuses on helping local businesses reach more people — especially those in their area.
Create your free business listing on PennySaver USA.
33. Ailoq
Domain Rating: 66
Monthly Organic Traffic: 300
Ailoq uses artificial intelligence to help businesses improve their marketing efforts. Ailoq’s AI business listings come with self-management advertising and SEO tools.
Create your free business listing on Ailoq.
34. Tupalo
Domain Rating: 51
Monthly Organic Traffic: 200
Tupalo allows businesses (especially those in hospitality, such as restaurants, hotels, cafes, nightlife, etc.) to create listings. The site’s social interaction element allows users to recommend businesses to others in their network.
Create your business listing on Tupalo.
35. Who Do You
Domain Rating: 73
Monthly Organic Traffic: 1.8K
Who Do You’s mission is to help users find the best local businesses to meet their needs. Aside from allowing you to make a listing, Who Do You aggregates recommendations shared on social media.
Create your business listing on Who Do You.
36. Good Firms
Domain Rating: 85
Monthly Organic Traffic: 308K
Good Firms is an excellent option if you offer B2B services. The site’s listings are often ranked based on thorough research to maintain credibility and reliability.
Create your business listing on Good Firms.
37. Clutch
Domain Rating: 90
Monthly Organic Traffic: 1.3M
Clutch is another directory website that lists businesses that service other businesses. Using AI, Clutch can quickly match your business with users looking for your services.
Create your free business listing on Clutch.
38. Scoot
Domain Rating: 75
Monthly Organic Traffic: 29.3K
Scoot is a directory website specifically for business owners in the United Kingdom. Scoot is part of the Scoot Network, which includes the newspaper website The Mirror (which has millions of daily readers), giving business owners more opportunities to get in front of more people.
Create your free business listing on Scoot.
39. Zumper
Domain Rating: 76
Monthly Organic Traffic: 381K
Zumper is a user-friendly online directory for real estate businesses. You can easily create listings and manage offers and rent applications.
Create your business listing on Zumper.
40. LocalStack
Domain Rating: 67
Monthly Organic Traffic: 1.5K
LocalStack is an online business directory that allows businesses to create listings so locals can find them. The site ranks business listings based on a social score (calculated from tweets, likes, and shares).
Create your business listing on LocalStack.
Benefits of Listing Your Business in Local Directories
As mentioned, the value of listing your business in directories isn’t about visibility in the directories themselves (although that’s certainly helpful); it’s more about building citations with your business information.
Here are some of the benefits of listing your business online. I know it’s a lot of work, but it’s endlessly invaluable.
1. Rank higher in local packs.
You’ve seen local packs before — they’re the groups of local businesses that appear when you make a location-based search, such as “pizza near me.” Here's an example of a local pack for that search in my area:
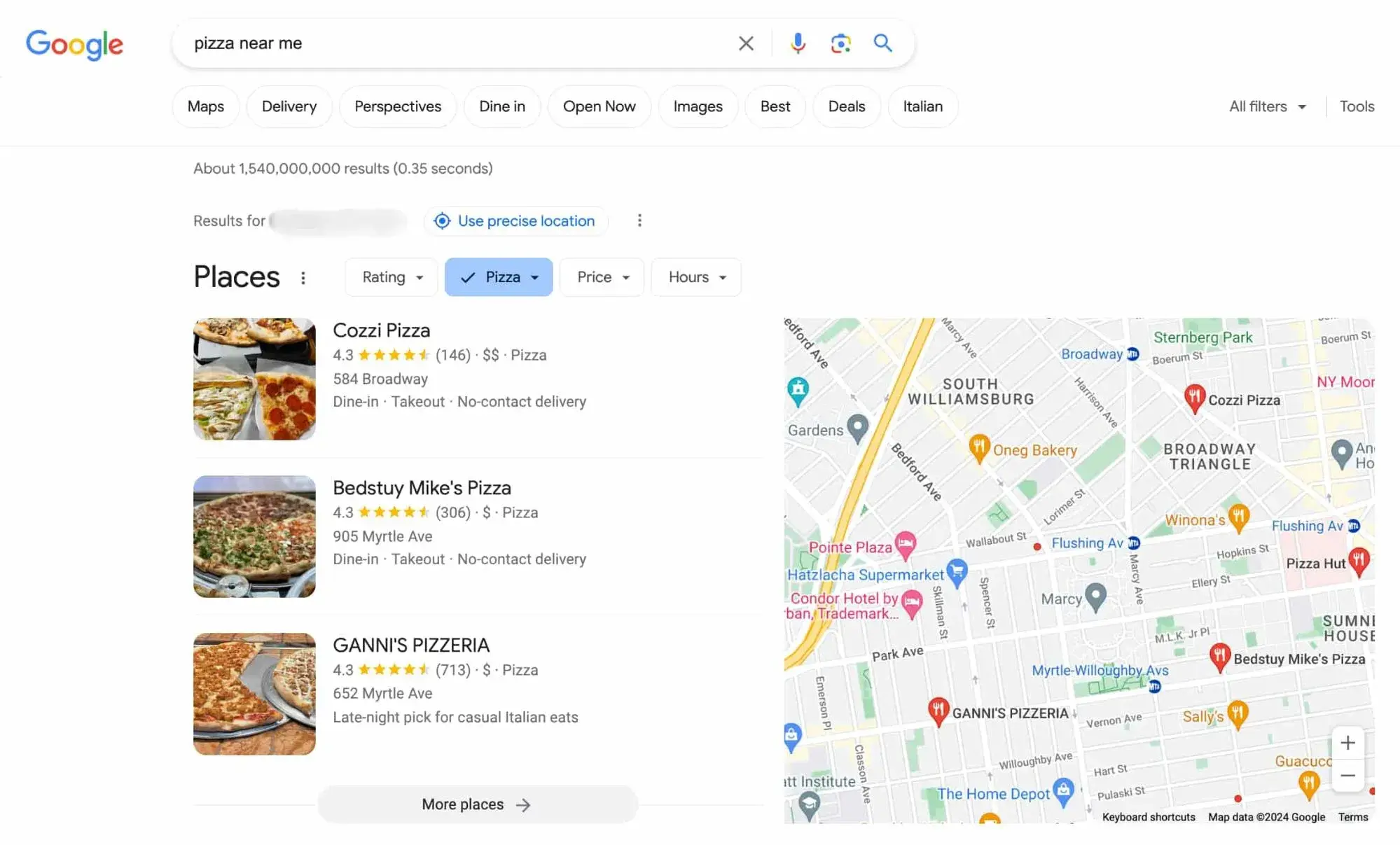
(Making a mental note to try these restaurants).
By listing your business in local directories, you’re reinforcing your company’s NAP information repeatedly. This constant and consistent record of citations (really, make sure your information is consistent!) can improve your chances of ranking higher in Google’s local packs.
You’ll, therefore, increase visibility and exposure in your area, which can result in more website visits and foot traffic.
2. Get white-hat backlinks to your website.
We can’t forget the big benefit: Backlinks. Most local directories let you link to your website, helping you bring in traffic from interested users. Some might be no-follow links, but it’s still worth the mention.
Most directories are authoritative websites with high domain authority or domain rating. So, by listing your business on these directories, you can indirectly increase your website's domain authority by getting a “vote,” so to speak, from an authoritative site.
In turn, getting backlinks or “votes” from directories can improve your search engine rankings and increase your website’s visibility and traffic.
3. Boost company legitimacy.
Creating business listings can also improve your legitimacy. Local directories are trusted sources of information, and being listed on these directories can boost your company's credibility and reputation.
Imagine if people looked up my business and the first SERPs were filled with random URLs that happened to share my business name. It definitely wouldn’t help me look established. But if the SERPs are all filled with listings from directories, users will feel like my company is real and worth their investment.
No matter what, being listed in local directories will improve your online reputation and increase your brand visibility.
Before You List Your Site
I’m sure you’re already excited about building citations by adding your business to relevant online directories on this list.
In addition to listing your site, you can improve your business’s visibility by making sure your website is up to snuff and optimizing your website for AI-driven search results.
Today, more people are using AI tools like ChatGPT and Perplexity to find answers and businesses. Even search engines like Google have started using AI in search results.
To see how well your website shows up in these results, use HubSpot’s AI Search Grader.
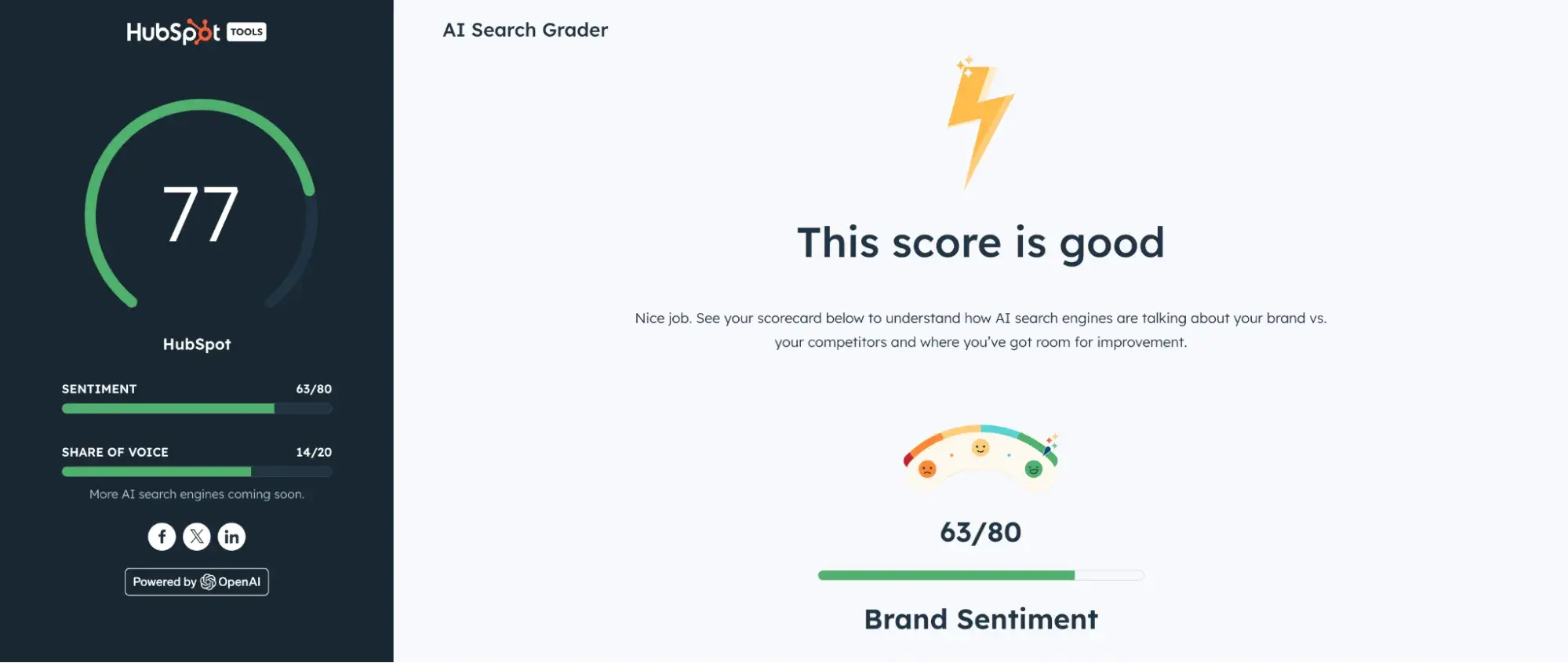
AI Search Grader helps you unlock valuable insights into your brand and pinpoint areas for improvement. You can also compare your performance with your competitors to see what works for your audience.
Grade your brand using AI Search Grader and Website Grader today — for free.
Add your local business to online directories.
Having a presence where potential customers might find you is critical to any local marketing plan. Building citations enhances your website visibility and adds SEO benefits.
Get started with SEO, add your local business to some of these business listings and directories today, and watch your business and customer base grow.
Editor's note: This post was originally published in March 2013 and has been updated for comprehensiveness.